Software
Blink Reaction: An Introduction to Google Tag Manager
When Google Tag Manager and Drupal work together, great things can happen. Both from a web developer's perspective and from a marketer's perspective. We'll take a look at how it all comes together.
Ken Rickard: DrupalCamp Colorado
I'll be heading out to Denver to give a Sunday keynote at DrupalCamp Colorado.
The theme of the event is "Enterprise Drupal," so we'll be diving in to what that phrase actually means for development firms.
If you're in Denver, please come on down and say hello.
Drupalize.Me: Guided Help Tours in Drupal 8 (sort of)
Pedro Rocha: Like & Dislike widgets for Drupal
Kristian Polso: How to make language switcher links link to frontpage in Drupal 7
NEWMEDIA: NEWMEDIA's Site Development Process (SDP)
When working in a team or in an environment where your code and systems are going to be used by people other than yourself, it is especially important that your site development process is clear, simple, and easy to understand. This, of course, is easier said than done when developing a complex Drupal site. However, when our developers, site-builders and themers are all on the "same page" with code organization and philosophy we are a more effective and efficient team.
After speaking with members of the Drupal community, we believe it is time to start a discussion on how to have a process in place so as to minimize the friction when developing in a team/cooperative environment. In an effort to deep dive into our process this article will be the first in a series of articles discussing our SDP.
A lot of our SDP revolves around how to organize your code so that a developer or site-builder can quickly on-board to a project and larger teams can work together with minimal down-time. The broad pieces of our SDP are:
- Everything is in code.
- Sites are built using install profiles and the install profiles have a specific directory structure.
- Install profiles use Drush Make to capture dependencies on external modules, themes, and libraries.
- Drupal migrate is used to populate test content during the development phase. (optional)
- Features are used to capture site configuration. (optional)
- While a site is in development all functionality must be present after a fresh site install.
- After a site goes live update hooks can be used to enable new functionality on the production site.
- Use a virtualized environment which mirrors production. (recommended)
What do you think?
Do you have an process expressed or implied? Leave comments below and lets keep this conversation going.
Last Call Media: Introducing Commerce Authorize.Net Card Present for Drupal 7

Commerce Authorize.Net Card Present is a new Drupal 7 module sponsored by Last Call Media that allows a Drupal website to accept payments by swiping a credit card through a USB credit card reader.
This module implements Authorize.Net's Card Present API to add a new card present payment method, and is based on the Commerce Authorize.Net module's widely used card not present implementation.
Developed to accompany Commerce Point of Sale (POS), another module sponsored by Last Call Media, Commerce Authorize.Net Card Present can be used in conjunction with Commerce POS to set up a fully functional Point of Sale system in Drupal 7.
For more information on how to install and configure this module, please visit Commerce Authorize.Net Card Present's project page.
Drupal core announcements: July 12-13, 2014 Asbury Park, NJ core sprint
On the weekend of July 12-13, 2014 the Central NJ Drupal Group held a core sprint focusing on the upcoming release of Drupal 8. The sprint was attended a great group of Drupal enthusiasts from Chicago, Montreal, New York, Virginia, and of course New Jersey. We took over the Cowerks coworking space in Asbury Park, NJ for the two days and it provided us a fun space to work the day and night away on a variety of issues.
With only seven beta blockers remaining at the time of our sprint, two were on the top of our list of items to work on. Fifteen people participated in person, and the group made progress on two beta blockers (leading to core commits) as well as many other issues.
See the full recap for more details on participants, issues worked on and completed, and a photo gallery.
Stanford Web Services Blog: Using Display Suite to provide field-level permissions
Have you ever wanted to show only selected information on a content type to anonymous users and more information to authenticated users? It turns out that you can use Display Suite to provide field-level permissions for an entity.
LevelTen Interactive: Did You Miss Our Webinar? Watch It Here!
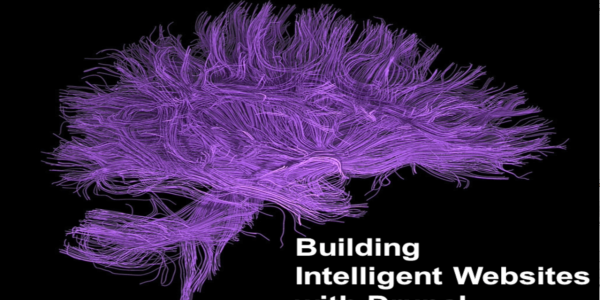
On Thursday, July 24th, we had a live webinar where we introduced Open Enterprise Intel. If you missed it, don't worry, we have the video right here as well as on YouTube available in HD.
... Read more
Appnovation Technologies: 3 Reasons Why Drupal Integrated Well With Other Tech

Acquia: New Cloud Features & UX Improvements
Acquia is constantly working to improve our service offerings, and that means cleaning up existing features and adding new ones that we know will make a big difference in people’s workflow for the better. Despite the scale of some of these improvements, they’re not always immediately visible at first glance, so I’ve taken a bit of time to highlight three recent ones.
DrupalCon Amsterdam: Growing the Community & the Project Through Grants, Scholarships, and Mentoring in Amsterdam
Grant and scholarship recipients have been selected for DrupalCon Amsterdam. We had a huge number of wonderful applicants, and selecting our grant and scholarship recipients was a challenge.
For applicants seeking grants, we focused on the importance of each candidate to the Drupal project and code as a whole. Scholarships, meanwhile, were awarded based on the impact or influence on the Drupal community and Drupal adoption that the person would have at their home region— though these were just a few of the many factors taken into account during the selection process.
We’re please to announce the following grant and scholarship recipients below:
Grant Recipients- Nathaniel (catch) Catchpole - United Kingdom
- Larry (Crell) Garfield - United States
- Dave (Dave Reid) Reid - United States
- David (David Hernández) Hernández Ruiz - Spain
- Dan (dcmul) Mulindwa - Uganda
- J Branson (j.branson) Skinner - United States
- Joël (joelpittet) Pittet - Canada
- Jose (Jose Reyero) Reyero - Spain
- Jeremy (jthorson) Thorson - Canada
- Kalpana (kgoel) Goel - United States
- Patrick (patrickd) Drotleff - Germany
- Brian (realityloop) Gilbert - Australia
- Ricardo (ricardoamaro) Amaro - Portugal
- Sébastien (SebCorbin) Corbin - France
- Shyamala (Shyamala) Rajaram - India
- Janez (slashrsm) Urevc - Slovenia
- Evgeniy (Spleshka) Maslovskiy - Belarus
- Tim (stpaultim) Erickson - United States
- Kristof (swentel) De Jaeger - Belgium
- Yves (yched) Chedemois - France
- Zsófi (zsofi.major) Major - Hungary
- Aldibier (aldibier) Morales - Colombia
- Alvaro (alvar0hurtad0) Hurtado - Spain
- Andrey (andypost) Postnikov - Russian Federation
- Carlos (camoa) Ospina - United States
- Luis Eduardo (edutrul) Yelaya Escobedo - Peru
- Grzegorz (grzegorz.bartman) Bartman - Poland
- Konstantin (konstantin.komelin) Komelin - Russian Federation
- Weber (Mac_Weber) Macedo - Brazil
- Ivan (rootwork) Boothe - United States
- Tanay (saitanay) Sai - India
- Shabana (Shabana Blackborder) Navas - India
- Tarek (tarekdj) Djebali - Tunisia
Congratulations to all of our grant and scholarship recipients! We'd also like to extend a big thanks to our selection team: Emma Karayiannis (UK) Bart Feenstra (NL) Mike Anello (US).
We’re excited to see all these great people at DrupalCon Amsterdam, and can’t wait to learn from them and make the project even better. When you see our grant and scholarship recipients around volunteering at the event or mentoring new sprinters, give them a high five for being amazing! And regardless of whether you’re receiving financial assistance or not, if you’re coming to DrupalCon, you can share you knowledge and help make Drupal even better too by signing up to become a mentor.
Growing the Drupal project can’t happen if our community doesn’t grow, too— and there’s no better way to help grow the community at DrupalCon Amsterdam than to give back by teaching new skills and ideas to basic, intermediate, and advanced Drupalers.
orkjerns blogg: Now running Drupal 8, in the most hipster way imagined.
It has been a weekend in the spirit of headless Drupal, front-end optimizations and server side hacks. The result is I updated my blog to Drupal 8. Since you are reading this, it must mean it is live.
First let's start with the cold facts (almost chronologically ordered by request lifetime):
- Varnish
- Nginx
- HHVM
- Drupal 8
- Mihtril.js
- Core REST module for all resources
Other front-end technologies used that does not directly relate to the request itself:
So, HHVM, huh?Yeah, that's mostly just a novelty act. There is no real gain there. Quite the opposite, I have added some hacks to get around some limitations.
HHVM does not work very well with logged in users right now, but works alright for serving anonymous content.
When I reload and look at the source code, there is no css loading. WAT?Yeah, I am just assuming you remember the styles from last page load. Also, I have made it an image to have a 1 HTTP request CMS, right?
No, really. How does that work?The real magic is happening by checking if you as a user already have downloaded my page earlier. If you have, I don't need to serve you css, as far as I am concerned. You should have saved that last time, so I just take care of that.
OK, so you use a cookie and save css in localstorage. Does that not screw with the varnish cacheGood question. I have some logic to internally rewrite the cached pages with a key to the same varnish hash. This way, all users trying to look at a css-less page with the css stored in localstorage will be served the same page, and php will not get touched.
What a great idea!Really? Are you really sure you have thought of all the limitations? Because they are many. But seeing as this is my personal tech blog, and I like to experiment, it went live anyway.
Give us the code!Sure. The theme is at github. The stupid cache module is at github. Please be aware that it is a very bad idea to use it if you have not read the code and understand what it does.
And since I am feeling pretty bad ass right now, let's end with Clint Eastwood as an animated gif.

Berliners blog: Showcase: Art market
The last months I was busy with a friends art project. Today I'm very happy to announce that it went public on july 15th and is doing good so far.
Jule, the founder of Port of Art, approached me last summer, asking if I could help her building an online market place for artworks. Working primarily as a freelance Drupal developer, knowing that her budget is tight and that she is certainly not the first one with this idea, I hesitated. But I gave it a thought and after several meetings I agreed. I liked the idea and I liked Jules approach, that is very trusting and positive without being naive. I like good people ;) She also gave me the impression of being able to value constructive input, even if it means to change previous ideas. That is a good feature in clients!
Basic ideas with a special flavorThe basic requirements were pretty simple:
- Content management for static content pages as well as for special content like the artworks that are sold on the site
- Search artworks by different filters
- Legal compliant checkout process
- Integration of external payment providers (limited to paypal for the moment being)
- Contact forms
- Multilingual content and communication
- Integration of social media
- Some map views for geo visualization
- SEO, customizability, ...
So far that was relatively straight forward and we all love Drupal for that.
But there were some special requirements too, that had a huge impact on my choice of modules to realize this with.
- Artworks don't integrate with a basic warehouse approach. Each one should be unique and can be bought only once. Therefor there was no need for a shopping cart either.
- Artworks can be bought for a fixed price or as an auction.
- Artworks under a certain price are not sold via the site, but instead the customer and the artist are put in touch directly and have to figure out the details independently of the platform.
- Artists should be able to upload their artworks, pay a fee to get them published and than manage the selling and delivery on their own.
- Artworks expire after a certain time that depends on the publishing fee that the artist is willing to pay.
- Once an artwork has been sold on the site, an additional fee has to be paid.
- Fully customizable e-mails
The main content is obviously the artwork. This is a node type with additional fields to represent attributes of an artwork. Then there are static pages, artschools, faqs and webforms. On the user side we have two frontend user roles for customers and artists that get enhanced using the Profile 2 module.
Additional considerationsThe situation that our development team was faced with: Small budget, tiny team (only 2 people), the project's concept still a little in the flux. The founder had no technical background or previous experience using Drupal but needed a customized shop system that she could actually manage after we finished the project and went on to other things. So one of the goals during development has always been to make things configurable. Special text at a certain page? Build a setting for that. A special criterion that controls logic during checkout? Don't hardcode it somewhere! Build a setting for that as it might change later and you don't want to change code for simple things. I love drupal for it's easy variable management and quick form building capabilities. Building an admin form to control certain behaviors takes rarely more then 10 minutes. Obviously there are things that you can't build that way, but when you can, do it. I feel much butter with it and the client loves it too because it gives him control.
Conception and development processOne of the things I knew before, but that got confirmed again: Communication is the key. The client has never did a web project before. That meant that certain good practices and workflow, concerning the development process as well as the final product, were not clear to her. So we (the designer and me) spend a good amount of time helping her figure out what was realistic and which compromises needed to be done in order to deliver the product without cost explosion or an exagerated time frame. Being honest and communicating potential problems early on, as well as the clients openness towards constructive input, was something that attributed a lot to the perceived quality of the development process. Including the client in the development and design decisions also allowed us to educate her on the technical aspects of the product and raise awarness about technical implications, making her see advantages and restrictions in different areas that she didn't consider in the beginning.
We didn't formalize the process, but we ended up with some kind of agile development with three distinct roles: Conception and design by the client, frontend by the designer and backend logic and architecural design by me. That worked very good for us.
First, there is Rules. A crazy wonderland for workflow configuration that amazes me every time I look at it. But I've almost never used it. Call me old fashioned, but when business logic or complex relations must be build, I prefer to build them on my own. I want as much logic as possible in the code, not in the database. So for all the power Rules provide, I still prefer not to use it.
Then there is Commerce. We have never build a real-world website with it, so our experience was very limited. We thought about it. Very seriously. Then we decided against it. From todays perspective that was probably an error. But given the special requirements we were afraid of having to spent too much time customizing and altering the workflow that commerce proposes. This was more of a gut feeling. And at the end I'm not sure it was the right decision. We ended up with conceiving and building a full fledged product management incuding the purchase logic and payment. The obvious advantage when you write something like this on your own, is that you have a lot of fine grained control about flow and design. But the price is pretty high considering the amount of time necessary. At the end we have a considerable code base that needs to be maintained. So next time, I hope I'll remember this an give commerce a more in depth examination regarding the potential for the problem at hand.
Crucial contrib modules / add onsIt's hardly necessary to mention, but we couldn't have build the site so easily without the usual candidates: Views, Webform, Better Exposed Filters, Address Field, CTools, i18n, References, Profile 2, Geofield, Global Redirect, Libraries, ...
The fantastic wookmark jquery plugin is responsible for the display of the central search component of the site. Our designer loves it!
Some modules that got born or advancedI build MEFIBS for this site. I had a need for that functionality before, but never quite as strong as this time, so I decided to solve it as a self contained module instead of hacking things together. Though there are some problems currently with a few new features that I added recently, it is already in production and doing pretty well. Have a look at the filter and sorting blocks on the artwork search page: . Two independant blocks without duplicating a views display or intensive custom form altering. That's pretty neat.
Hopefully the jQuery Update module will also profit. During development I ran into issues with the admin version feature introduced here: https://www.drupal.org/node/1524944. I wrote about it in jQuery version per theme. This resulted in a feature patch that is currently on a good way to get committed soon.
I also found a bug in the PayPal for Payment module: https://www.drupal.org/node/2052361 that will hopefully get fixed soon.
Another module I find myself using often is my sandbox module Mailer API. It's a bit cumbersome to use as a developer, but for the client it's perfect. She can customize practically every mail that will be send by the system. It's all on a single configuration page and supports multilingual setups. A test mail feature is also included to see what mails will look like. And a batch mailer that the client often uses to address a bunch of people. It's like very easy home made promotional mails in a consistent look and feel. Made the client happy.
For frontend eye candy we have build a jQuery plugin that is responsible for the collapsible checkbox filter elements in the left side bar.
Some module discoveriesDuring the work on www.port-of-art.com I found some modules that I didn't know before.
The Form API Validation module allows you to simplify validation rules in custom forms, using predefined validation rules. And you can also add your own rules which we used for the price entry validation needed when artists publish their artworks.
The Physical Fields module provides fields for physical dimensions and for weight attributes. That was exactly what we needed for physical goods. It saved us the time to configure fields in field collections.
ConclusionAt the end of the project I can say, that everyone involved has had a good and productive time and enjoyed the process and the result. The client is happy for all the things she can do with the site. Now she can concentrate on managing business and extending marketing. The designer was happy. Even if some of the design decisions might not have been the best ones looking at the requirements profile from today. I feel positive though that the system fully matches the clients expectations and that it'll be a valuable tool for developing her business. If the site manages to establish itself, it's more than probable that we would rebuild the system, at least some substantial pieces like the shop component.
We as the site builders are happy too. We feel that we have done a good job and that we managed to keep resources and expectations in balance. I would do it again, which always feels like a good measure.
Category: Drupal Planet7.xCode Drop: Drupal 7 WYSIWYG Editors Done Right
It's fair to say, on a fresh install the content authoring experience in Drupal needs improvement. WYSIWYG editors are often criticised for various reason such as the ugly HTML they are known to generate or the power they give users to mess up typography. While these are valid criticisms, there is definitely a right and wrong way configure these editors. Doing things the right way will empower users while keeping them safe from nasty pitfalls. Note: this guide assumes you're already familiar with a typical Drupal WYSIWYG setup.
Provide a true WYSIWYG experienceIt's important that a WYSWIYG editor represents exactly what appears on the front-end of your website. While it seems obvious, it's easy to ignore and has a big impact on a user's experience.
Our WYSWIYG stack is:
Drupal.org Featured Case Studies: Concern Worldwide - Mobilisation & Usability
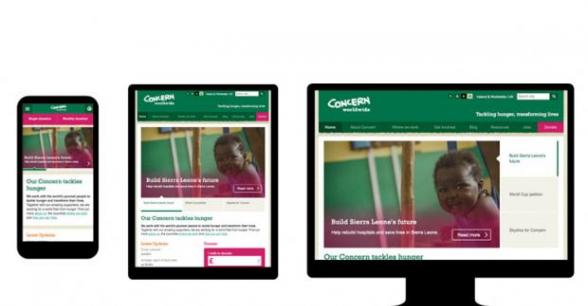
Concern Worldwide is a leading international humanitarian organization dedicated to tackling poverty and suffering in the world’s poorest countries. Their main website, www.concern.net, plays an important role in their fundraising process. It enables people from around the world to donate towards specific campaigns and ensure that their money is distributed to where it’s needed most.
SystemSeed has the wonderful opportunity of partnering with Concern in leveraging Drupal to power the Concern Worldwide platform for a number of years spanning all the way back to the days of Drupal 5. This particular site was upgraded to Drupal 7 in 2012 as part of a large platform refactor which aimed to consolidate all of Concern’s Drupal websites under a single common platform. We wrote about the transition from standalone sites in this case study.
Since then, we have been leading a large project to bring full responsiveness and optimisations across a wide spectrum of devices to all sites on that platform. Today (July 3, 2014) sees the completion of this project with the rollout of a fully responsive and adaptive theme layer, catering for its widest audience ever. In this case study, we’ll look at some of the components of this project, the processes, the challenges, the successes, and lessons learned along the way.
LightSky: NavBar - The Next Step in Drupal Navigation
So I am not kidding NavBar is literally the next step in Drupal navigation, it is being used in core for Drupal 8. This is great news because not only does it mean that the Drupal 8 core will contain some much needed improvements to the administration navigation scheme. Back end user improvements like this are perhaps the thing that makes me most excited about what Drupal 8 is bringing to the table. Lets look a little bit at NavBar.
What You Get
Pretty simply put NavBar gets you a responsive administration toolbar for your Drupal users. It really isn’t going to do anything for what your visitors see, but your content creators, site administrators, and even site builders will see this as a much welcomed change. NavBar is first and foremost completely responsive, and for those of you who use the traditional Drupal administration toolbar on your mobile phone oh boy are you excited. The standard Drupal 7 install, not to mention Drupal 6, doesn’t offer the most mobile friendly administrative experience. NavBar helps resolve this. NavBar also offers a more flexible navigation option. You are able to use NavBar at the top of your site above the header, or as a sidebar on the left hand side. The customization of the tool, really helps set it apart.
Not only is the mobile experience improved, but there is a much cleaner and professional looking image presented than the Drupal 7 administration menu. Though this might not seem like much, for those of us who build Drupal sites for clients this is a big deal. Image is everything, and it is tough to sell Drupal’s out of the box usability against WordPresses out of the box usability. We have a lot of admin usability improvements in our standard Drupal installation to help combat this, but now NavBar is another one. Users almost expect clean and friendly design, and now they can get it.
Installation
I am not going to lie, NavBar in its current state is a bit of installation work, but most people should be able to figure it out if they have a little understanding for how Drupal is structured.
The first step for me is downloading and installing the project. I think that drush is the best tool for installing and enabling projects like this, but particularly for NavBar I suggest installing the project before moving to some of the other steps. The reason is that once the project is installed and enabled it will put some indicators on your /admin/reports/status page that can really help you troubleshoot in the next steps.
Once the NavBar module is enabled, you can visit the site’s status report using the path above and notice that there are a three statuses now associated with NavBar, and this is where the fun comes in. NavBar requires the installation of three libraries (Modernizr, Backbone, and Underscore), and you may have them already installed, or at least some of them. Using the status page at this point will help you find out if you have them already installed and ready to run, or whether you need to install them.
If you find that you need to install them, the process isn’t all that complicated, there are some helpful guides on the project page that will point you in the right direction. Or give us a shout we would be happy to help. Essentially it is a matter of downloading the libraries, or cloning their respective repositories, and moving them to your libraries folder in the Drupal installation. The Modernizr library requires you to follow a link and download a specific minimized version of the library but there are specific instructions to follow on the project page to help guide you here, so I won’t reinvent the wheel here. The instructions are pretty thorough, and relatively simple.
Once you have the libraries installed you can disable your regular administration toolbar and you are off and running. If you follow those steps and still aren’t having any luck, the site status report is the best place to look. Most likely it is an error with the libraries that were installed, and that report will point you to which library is causing trouble, and maybe even what the problem is.
We have fallen in love with NavBar, and it has started making a huge impact on our clients and how well they like using Drupal. We highly suggest you use it.
For more tips like these, follow us on social media or subscribe for free to our RSS feed and newsletter. You can also contact us directly or request a consultation.