Software
Matthew Saunders: Anatomy of a Drupalcamp - The Tools
I've been involved with Drupalcamp Colorado since 2007. Sometimes I've had a significant role, other times I've taken a bit more of a back seat. I was also pretty heavily involved in Drupalcon when it was in Denver. Over the last 8 months or so, I've had quite a bit more insight into the Cons themselves through my interaction with the Drupal Association. This last year I've been the project manager for Drupalcamp Colorado 2014. This has left me with with some personal insights that might help others wanting to run a camp.
drupaldrupalcamp coloradohow to do a campStanford Web Services Blog: Agile Project Management and its flavors: where does Scrum end and Kanban begin?
Agile has become a big buzz word recently in the project management world. In this post, I'll try to clarify where some of the lines are being blurred between terms like Agile and Scrum, and what some of these terms actually mean.
Keep in mind while reading this that I am primarly trained in Scrum, so my descriptions of other Agile methodologies are only decently informed. :)
What is Agile Project Management?From the ever-present wikipedia:
Drupal 8 and iOS: REST Export for Drupal 8 View
Hi! Drupal Community.
As we all are working very hard for upcoming major release I am feeling excited to write this. This blog is all about REST Export feature of Drupal 8’ view. Let me first brief you about what is the REST api and than I will explain you how REST Export is important for building custom REST api for your Drupal 8.
REST (Representational State Transfer) is a web service architecture to expose your resources to outer world. It follows http architecture to expose the resources of your web site i.e you have GET, POST, PATCH,DELETE etc methods to manipulate resources with out web interface. In context to Drupal , a node will have GET method that will require node id and it will return you details related to that node in XML, JSON or HAL + JSON format. If you have ever used REST api for some famous web services like twitter, facebook then you will find those API very well defined for example GET on /status will give you current status details and GET on /status/comments will give you comments posted on your current status. In context to Drupal we should get node details with GET on /node/{id} and comments on particular node with GET on /node/{id}/comments. In Drupal 8 we have first REST endpoint but we don’t have second. Drupal has opt for different approach. In Drupal you can use contextual filters on view to filter content as per your parameters passed in URL . The same concept we can applies to REST Export . We can attach a REST Export to a view that only show comments with contextual filter with nodeID as parameter. So the rest export will return XML or JSON for comments related to particular node.
Here is a screenshot for it.

We can use REST Export with taxonomy_terms for particular vocabulary as parameter. This concept will make Drupal 8 REST api robust and it also provides good example where view modules and REST module works to gather to provide developer flexibility to develop custom REST api for their Drupal site.
Tags:Drupal core announcements: Drupal 8 alpha 14 on August 6th
The next alpha for Drupal 8 will be alpha 14! Here is the schedule for the alpha release.
August 3rd-5th Only critical and major patches committed August 6th, 2014 Drupal 8.0.0-alpha14 released. Emergency commits only.(Note that there are two concurrent Drupal 8 sprints Aug. 7-10, so we aren't planning to have the usual "disruptive patch window" following this alpha.)
Appnovation Technologies: 3 Reasons to use Drupal for Enterprise Online Portal Dev

Dcycle: New Drupal 7 project checklist
I had this checklist documented internally, but I keep referring back to it so I'll make it available here in case anyone else needs it. The idea here is to document a minimum (not an ideal) set of modules and tasks which I do for almost all projects.
Questions to ask of a client at the project launch- Is your site bilingual? If so is there more than one domain?
- What type of compatibility do you need: tablet, mobile, which versions of IE?
- How do you see your post-launch support and core/module update contract?
- Do you need SSL support?
- What is your hosting arrangement?
- Do you have a contact form?
- What is your anti-spam method? Note that CAPTCHA is no longer useful; I like Mollom, but it's giving me more and more false positives with time.
- Is WYSIWYG required? I strongly suggest using Markdown instead.
- Confirm that all emails are sent in plain text, not HTML. If you're sending out HTML mail, do it right.
- Do you need an on-site search utility? If so, some thought, and resources, need to go into it or it will be frustrating.
- What kind of load do you expect on your site (anonymous and admin users)? This information can be used for load testing.
- If you already have a site, should old paths of critical content map to paths on the new site?
- Should users be allowed to create accounts (with spam considerations, and see if an admin should approve them).
Here is what should get done in the first Agile sprint, aka Sprint Zero:
- If you are using continuous integration, a Jenkins job for tracking the master branch: this job should fail if any test fails on the codebase, or if quality metrics (code review, for example, or pdepend metrics) reach predefined thresholds.
- A Jenkins job for pushing to dev. This is triggered by the first job if tests pass. It pushed the new code to the dev environment, and updates the dev environment's database. The database is never cloned; rather, a site deployment module is used.
- An issue queue is set up and the client is given access to it, and training on how to use it.
- A wiki is set up.
- A dev environment is set up. This is where the code gets pushed automatically if all tests pass.
- A prod environment is set up. This environment is normally updated manually after each end of sprint demo.
- A git repo is set up with a basic Drupal site.
- A custom module is set up in sites/*/modules/custom: this is where custom function go.
- A site deployment module in sites/all/modules/custom. All deployment-related code and dependencies go here. A .test file and an .install should be included.
- A site development module is set up in sites/*/modules/custom, which is meant to contain all modules required or useful for development, as dependencies.
- A custom theme is created.
- An initial feature is created in sites/*/modules/features. This is where all your features will be added.
- A "sites/*/modules/patches" folder is created (with a README.txt file, to make sure it goes into git). This is where core and contrib patches should go. Your site's maintainers should apply these patches when core or contrib modules are updated. Patch names here should include the node id and comment number on Drupal.org.
- views
- context
- strongarm
- admin_menu
- markdown
- transliteration
- globalredirect
- redirect
- config_builder, almost always useful to create a single form allowing clients to change site variables.
- context
- features
- logintoboggan
I normally create a custom development module with these as dependencies:
- diff
- devel
- realistic_dummy_content_api (part of realistic_dummy_content)
- coder
- context_ui (part of context)
- views_ui (part of views)
- devel
- devel_themer
- masquerade
- search_krumo
- simpletest_turbo
- devel_generate (part of devel)
- config_builder_ui (part of config_builder)
- Design a custom 404, error and maintenance page.
- Path, alias and permalink strategy. (Might require pathauto.)
- Think of adding revisions to content types to avoid clients losing their data.
- Don't display errors on production.
- Optimize CSS, JS and page caching.
- Views should be cached.
- System messages are properly themed.
- Prevent very simple passwords.
- Using syslog instead of dblog on prod
Most shops, and most developers, have some sort of checklist like this. Mine is not any better or worse than most, but can be a good starting point. Another note: I've seen at least three Drupal teams try, and fail, to implement a "Drupal Starter kit for Company XYZ" and keep it under version control. The problem with that approach, as opposed to a checklist, is that it's not lightweight enough: it is a software product which needs maintenance, and after a while no one maintains it.
Tags: blogplanetModules Unraveled: 113 - Updates on the WalkHub project with Kristof Van Tomme - Modules Unraveled Podcast
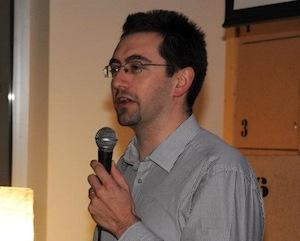
- You’ve been on the show before to talk about walkthrough.it, but some things have changed since then. So, can you give us an overview of what Walkhub is?
- What are walkthroughs?
- What is your pricing model on Walkhub?
- You’re in the process of a second Indiegogo campaign, but what was the first one for?
- How did your first Indiegogo campaign go?
- What is the current Indiegogo camaipn for?
- What’s the status on that?
- Why are you doing another campaign? Why aren’t you out of BETA yet? What’s the story there?
- You just did an AMA on Reddit yesterday. How did that go?
- What were some common questions? Or ones that stuck out to you?
Phase2: Amazing Design Through Empathy
The difference between a good product and an amazing one boils down to one thing: Empathy. Developing an understanding of your users that is so deep that you can feel what they feel enables you to design products and experiences that will truly resonate with your users.
Through illustrative and entertaining examples, I will take you on a tour of the the highs that are achievable through empathic design, and some of the depths that designers sink to when they design without empathy. You’ll learn how to activate the empathy that is already within you, and how you can use that power to improve all aspects of your product design, from requirements gathering to user research, and everything in between.
Join me for my presentations on this topic this week:
Drupal Capital Camp & Gov’t Days
Wednesday, July 30 from 11:15AM – Noon in room F1/F2
Design4Drupal Boston 2014
Saturday, August 2 from 3:30 – 4:30pm in room 141
Code Karate: Drupal 7 Panelizer Module

The Drupal 7 Panelizer Module allows you to panelize (or use panels) for any entity type on your Drupal 7 site. This allows you to change the layout of a node page, user page, or any other type of entity that you can think of. Although it's a little more complicated to set up, the Panelizer module allows you to set up a slimmed down panels interface for other site managers to use.
Tags: DrupalPanelsDrupal 7Site BuildingDrupal PlanetForum One: Double the Fun: DC Drupalists Unite!
What could be more fun than summer camp? A Drupal summer camp, of course. And what could be more fun than a Drupal summer camp? Why, two Drupal summer camps, of course – merged into one!
This week we’re witnessing the unity of two long-awaited DC Drupal events with the joining of CapitalCamp and Drupal 4 Gov into a single event – CapitalCamp and Gov Days – and we’re enthused by all the possibilities that will come from having our local Drupal friends all in one place!
On Your Mark, Get Set…This year we’re doing something brand new at CapitalCamp – we’re sprinting! That’s right, Forum One is hosting a Coder Lounge and Code Sprint room (Rooms C1 & C2) for fellow Drupalists to collaborate and bang out code together. This dedicated room will be available throughout CapitalCamp and Gov Days for developers to meet, greet, and collaborate on Drupal 8 code sprints. Developers will be convening in the mornings and afternoons to introduce sprints, identify issues, and get each other up and running…er, sprinting. Whether you’re new to code sprints or a seasoned veteran, come join us and contribute to the future of Drupal!
Expert SessionsOur all-star team is proud to be presenting five sessions to the DC Drupal community this year!
On Wednesday, Dan Mouyard presents on Advanced Theming Tactics, covering how to follow the Drupal 8 CSS Architecture Guidelines while theming a Drupal 7 site, including:
- Controlling Drupal’s markup
- Creating custom layouts
- Organizing your Sass partial structure
- Using SMACSS to write maintainable CSS
Later in the day, William Hurley will be demystifying acronyms and bureaucratese with his session on Deploying Secure Drupal Sites, providing a roadmap for developers and IT managers to meet government security obligations while laying out specific issues that might be encountered, and how to overcome them.
On Thursday afternoon, Michael Rader, Sarah LeNguyen, and John Schneider are excited to present Hot Planet, Cool Site: Relaunching GlobalChange.gov – the inside story of the design and relaunch of this major website under the U.S. Global Change Research Program (USGCRP) that focuses on assessing the impacts of climate change in the United States. Relaunching GlobalChange.gov was a big project that garnered big praise from the national press (and even the White House!), so we’re thrilled to be presenting on the site with our client at USGCRP for the first time.
And also on Thursday, Kalpana Goel will join with William Hurley to present a session on Routing in Drupal 8 to explain the many parts of routing, how to convert from the Drupal 7 menu system to the Drupal 8 routing system, as well as defining local tasks, local actions, and contextual links in Drupal 8.
But that’s not all, because on Friday, William returns for a CapitalCamp threepeat to present his final session of the conference: Automate All The Things – addressing why you need to be using automation, the tools you can use to make your development faster, easier and less error-prone, and common pitfalls that you might encounter along the way.
Seeing RedAs at DrupalCon last month, you’ll find Forum One staff in the hallways, sessions, and BoFs wearing our red shirts. Among our other attendees this week:
- Cindy Lopez-Yusuf and Ned Baker will be mingling with Drupal job hunters at Wednesday’s noontime networking mixer.
- Kerry Thell, Andrew Cohen, Riccardo de Marchi Trevisan and Eric Davis will be representing our Business Development team throughout the week.
- Keenan Holloway and Martin Franzini will round out our tech and UX folks on site.
Once again, we’re excited as always to be actively participating in our hometown Drupal camps and are looking forward to sharing our knowledge, meeting new Drupal friends, and learning about new projects for us to explore.
Will you be at CapitalCamp and Gov Days this week? Stop by the Forum One Coder Lounge to say hi, or drop us a line at marketing@forumone.com – we’d love to meet up with you at Camp this year!
Janez Urevc: Progress of Disqus project in GSoC 2014
For more info about the project and it's progress see the post on groups.drupal.org.
DrupalCon Amsterdam: Become a Mentor at DrupalCon Amsterdam
From volunteering your time at events to making a donation, there are plenty of ways to give back to the Drupal project - but by and large, one of the most important things individual Drupalers can do is donate their expertise and become a mentor.
Currently, we have only 24 mentors signed up, and we need 40 mentors to make DrupalCon Amsterdam a success. We’re anticipating several hundred individuals sign up for to join the sprint on Friday and mentorship is a great way to help people new to contributing learn Drupal and, eventually, contribute back valuable time, resources, and code to the project.
To become a mentor, click here to sign up. We need mentors for all levels of Drupal expertise, from teaching absolute Drupal beginners to assisting advanced users how to navigate the Drupal.org issue queue.
Need a ticket to attend?There are a limited number of free DrupalCon ticket coupons available for people who sign up to mentor, and the deadline to sign up and request a ticket is Friday, 1 August. Don't miss out on an opportunity to help others and get your ticket sponsored!
We’re looking forward to the Amsterdam Mentored Code Sprint and the First-Time Sprinter Workshop. We hope that you’ll join us there!
--
Cathy Theys (YesCT)
Brian Gillbert (realityloop)
Ruben Teijeiro (rteijeiro)
DrupalCon Amsterdam Sprint Leads
Drupal Association News: Announcing Our Newest Staff Members
We’re thrilled to announce the addition of four new staff members to the Drupal Association team. Please help us give a warm welcome to Oliver Davies, Archie Brentano, Phillip Bulebar, and Ryan Aslett!
Oliver (opdavies) started with the Drupal Association in May of this year as a Drupal.org Developer. He has been active in the Drupal community for several years, has contributed numerous patches and modules, and prior to working with the Drupal Association, he contributed to the accessibility of the project. Since joining several months ago, Oliver has already made tremendous contributions to the Association and has seized the opportunity to give back to the community in any way he can.
Archie Brentano (isntall) is the Association’s new DevOps Engineer. Previously, Archie worked as a Multnomah County System Administrator, focusing on enterprise Drupal sites on Amazon Web Services infrastructure. Archie will be concentrating on the infrastructure side of Drupal.org, and has joined the organization because he was impressed by the Drupal community and saw a perfect opportunity to learn more about Drupal and become better involved.
Phillip Bulebar (pbulebar) comes to the Drupal Association with a long and successful track record in marketing and web content management. As the Association’s new Content Manager, Phillip will be creating and optimizing content on Drupal.org to help ensure it meets the needs of visitors. Prior to joining the Association, Phillip held management roles for companies including Nike, Nautilus and other specialty retailers, with much of his focus on creating, delivering, analyzing and optimizing digital content.
Ryan Aslett (mixologic) is joining the Drupal Association as the organization’s first QA Engineer. Previously, Ryan worked as a freelance full stack Drupal developer in the Portland, Oregon area; he has a wide variety of experience in everything from working with Perl to engineering composting toilets in Ecuador and Colombia. At the Association, Ryan will be improving BDD tests for Drupal.org websites, and we’re thrilled to welcome him on board.
Please help us give a warm welcome to our four newest staff members. We’re thrilled to have them on board and know they’ll do great things for the Drupal community.
Drupal Easy: Drupal Web Developer Career Series Post 4: View from the Summit
This is final installment of our four-part blog post series that encapsulates the advice, tips and must-do elements of career building in the Drupal Community from the panel of experts collected for DrupalEasy’s DrupalCon Austin session; Drupal Career Trailhead; Embark on a Path to Success. It will be listed with other career resources for reference at the DrupalEasy Academy Career Center.
Drupal Easy: DrupalEasy Podcast 136: Wolves (Jason Smith - Weather.com)
Jason Smith (Silcon.Valet), Solutions Architect for Mediacurrent, joins Mike, and Ryan to talk about one of the highest-trafficked sites in the world re-launching on Drupal: weather.com. Other topics discussed include the Acquia CEO’s recent Reddit AMA, sprint nutrition, and Damien McKenna.
Freelock : Selling a Drupal-based product
Midwestern Mac, LLC: Moving on to Acquia
I wanted to post this here, since this is more of my sounding board for the Drupal community, but the details are on my personal blog: starting October 6, I will be working for Acquia as a Technical Architect in their Professional Services group!
What does this mean for this site/blog, Hosted Apache Solr, and Server Check.in? Not much, actually—they will continue on, likely at the same pace of development they've been for the past year or so (I'll work on them when I get an odd hour or two...). I am still working on completing Ansible for DevOps, and will actually be accelerating my writing schedule prior to starting the new job, since I'll have a little wedge of free time (a.k.a. unemployment!) between Mercy (my current full-time employer) and Acquia.
Blink Reaction: An Introduction to Google Tag Manager
When Google Tag Manager and Drupal work together, great things can happen. Both from a web developer's perspective and from a marketer's perspective. We'll take a look at how it all comes together.
Ken Rickard: DrupalCamp Colorado
I'll be heading out to Denver to give a Sunday keynote at DrupalCamp Colorado.
The theme of the event is "Enterprise Drupal," so we'll be diving in to what that phrase actually means for development firms.
If you're in Denver, please come on down and say hello.