Software
KYbest: Exporting image field defaults in D7
We all love image fields' defaults: it's so easy to have a hero image for a product or a colleague's profile even when the editor does not provide one, with all the niceties such as displaying it with various image styles in a list, in the teaser or on the actual page. We all love Features module as it allows us to export Drupal 7 content types with all its settings.
Modules Unraveled: 114 What PHPStorm brings to Drupal Developers with Maarten Balliauw - Modules Unraveled Podcast
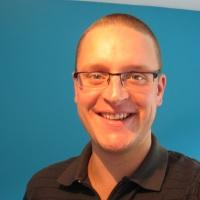
- I’ve recently started using Sublime Text, how would you compare PHPStorm to other text editors?
- What is an “IDE”?
- What are some of stand out features of PHPStorm?
- Where can people find out more about how to use the features of PHPStorm?
- What is the pricing structure?
PHPStorm and Drupal
* What integrations does PHPStorm have with Drupal 7, right now?
* What will PhpStorm do for Drupal 8?
- Marc Drummond
Really enjoy using @phpstorm. Always interested in learning how to get more out of my use of it.
Drupal Association News: What the Association Board Does, and Why You Should Run!
One of the things that sets the Drupal community apart from other open source projects is our big, amazing, and very diverse community. The Association board is structured to help represent our community with two community elected seats. After a an update to our bylaws this May, we now elect one board member per year to a two-year term. These seats are open to all community members, and we need you to fill them! Our next election will be held in early 2015, and we need your help finding great candidates.
As we shared in the 2013 election wrap up presentation at the DrupalCon Prague public board meeting, we have a couple of challenges when it comes to public elections. The first is fielding a diverse candidate pool. We had some great candidates, but very few women, people of color, or candidates from outside the US. In our next election, we'd like to see more diversity in the candidate pool. Secondly, we have very low turn out for the elections. Anyone with a Drupal.org user account created before nominations open and with at least one login in the last year can vote. Yet, we had just 668 votes in the last election - not even a full percent!
So now we're on a mission to make sure that the community understands the role of the board in the Drupal Project, the work that the board undertakes, and what it takes to serve on the board yourself. On Tuesday, 5 August, we held a webcast that outlines what the board does, how the elections work, and how to run. You can watch the whole thing here, and check out some of the key points, below.
What does the Board Do?Association board members, like all board members for US-based organizations, have three legal obligations: duty of care, duty of loyalty, and duty of obedience. In addition to these legal obligations, there is a lot of practical work that the board undertakes. These generally fall under the fiduciary responsibilities of the board and include:
- Overseeing Financial Performance
- Setting Strategy
- Setingt and Reviewing Legal Policies
- Fundraisng
- Managing the Executive Director
To accomplish all this, the board meets each month for two hours, and comes together for the two-day retreats, usually scheduled to coincide with the North American and European Cons as well as one January or early February meeting. As a board member, you can expect to spend a minimum of five hours a month.
How do Nominations and Elections Work?The first elections were held in 2012 after a lengthy community discussion was held to determine the specifics of who should be able to run, how voting would work, and who would be eligible to vote (among other details). The planning discussion that surfaced most of these issues is a great background read. The result is a nomination and voting process that is still in use today.
Nominations are only accepted as self-nominations in our elections. You may not nominate another person. We accept those nominations during a short (two to three week) window, and after much outreach into the community. To nominate yourself, you need only complete a short form that asks for information such as your bio, your interest in running, what makes you a good candidate, as well as a photo and contact information.
For voting, we use the Instant Run Off method. The method of voting ensures that whoever wins is supported by a majority of voters. Voters rank candidates according to their preferences, and do not have to rank every candidate to complete their votes. Voting will be open for a week to two weeks, and any individual with a Drupal.org account before nominations open and who has logged in at least once in the prior year may vote.
Oh, and in case you're wondering, we use the Election Module to make this all happen.
Who Can Run? How do I Run? What do I do?Anyone can run for the board, and we welcome all candidates. However, we do want to point out that serving on the board is very different than many of the other opportunities to contribute to the Drupal community. The board works at a strategic level, not a tactical one, and is often thinking one to three years out at a time. We want new board members to find ways to contribute and have a voice as quickly as possible, so we recommend that you have (or that you find) experience with any of the following types of areas:
- Other board experience
- Reading financial documents
- Drupal community committee experience
- Strategic planning
- Policy development
However, I want to stress that NONE of these are REQUIRED to run for or serve on the board. There are many great and free resources at BoardSource and the Bridgespan Group about board service and board skills. And because elections are not for six months, you have plenty of time to get some reading under your belt!
All completed candidate self-nomination forms are published at the end of the nomination period. We call this phase "Meet the Candidates" and community members will be able to ask questions of you on your candidate profile page. Additionally, we will set up several webcasts for candidates to address community questions live, in a variety of time zones.
When are the next elections?In prior years, we held elections in the fall. This was difficult because it meant that most of the recruitment and meet the candidate work was done in August, when many humans in the northern hemisphere are on vacation. We've switched to a beginning of the year format which allows us to elect and ratify a candidate with plenty of time to get the onboarded and prepped to attend the DrupalCon North America retreat. Here is the schedule for the next election:
- Community Discussion (August/September 2014)
- Technical Setup (December/Jan)
- Nominations (February 1-20)
- Meet the Candidates (Feb 21 - March 6)
- Voting (March 9 - 20)
- Ratification & Communication (March 25)
Have more questions? Never fear! We are always happy to answer them. Leave them in the comments or send me an email.
Symphony Blog: FAQ Field vs FAQ module in Drupal
We had been always using FAQ module to create Frequently Asked Questions for any of our projects. But on our latest theme, BizReview, we switch to a new module, FAQ Field.
FAQ is a classic module, it is there since Drupal 6. So when we have to build a FAQ section, using this module is a no brainer. This is the FAQ module in action on our Velocity theme.
Advomatic: Takeaways, besides cheese, from DrupalCamp Wisconsin
Everybody knows that Wisconsin is home of America's largest water park, the world’s largest barber pole, the world’s largest penny, and the world’s largest talking cow. Another thing to know is that Wisconsin is also home to a very active Drupal community and July's excellent DrupalCamp!
It’s been a few years since my last Drupal conference, so DrupalCamp was a great opportunity to catch up on new best practices and get a little reassurance that Drupal 8 isn’t something I should fear as a developer.
Here are the highlights from a few sessions I attended:
Using Drupal for Government and Open Data ProjectsGovernment sites pose unique challenges, and Sheldon Rampton explained how Drupal can be leveraged to accommodate for these issues if you plan well. Sheldon focused on hosting, architecture, standards, process and project management, and I definitely appreciated him referring to developers as “the talent” instead of “the resources.”
You can see his slides here.
In a related talk, Janette Day gave us an overview of DKAN, a Drupal platform for handling open data. Using Drupal for open data projects makes sense - open data should be open source, and Drupal allows non-technical users to manage content. Drupal can also help an organization modernize antiquated systems and escape from expensive software licenses.
You can check out my notes here.
Other Cool ProjectsDavid Snopek gave us a run-down of Drupal distributions with a focus on Panopoly, which adheres to UX principles that really streamline the content-creating experience. I haven’t used Panopoly on a project myself, but I’d absolutely give it (or some of its components) a spin next time I’m tasked with hooking up a WYSIWYG or making in-place editing more client-friendly.
And if you’ve ever run into trouble on your site with managing dependencies among your modules, go check out Jim Rath 's module dependency grapher. Using a custom Drush command, the dependency analyzer will inspect your modules and generate a chart which illustrates the dependencies between them.
You can check out everything else from DrupalCamp that I bookmarked up at https://pinboard.in/search/u:hey_germano?query=drupalcampWI2014
Forum One: Routing in Drupal 8 (a Capital Camp Session)
Last week, our team participated in the first Capital Camp and Gov Days, graciously hosted at the National Institutes of Health in Bethesda, Maryland. It was a great event and we would like to thank the organizers and volunteers for making it such a success!
On the second day of the conference, William Hurley and I presented a session on Routing in Drupal 8, reviewing what changes are being made in Drupal 8 and why these changes were necessary. One of the major variations in the new system compared to Drupal 7 is the alteration of the routing process. In Drupal, a route is a mapping between URL paths, their corresponding pages and access callbacks. In Drupal 7, these routes are defined by the hook menu, using a 1:1 path to route. Drupal 8 has done away with hook menus altogether, and we now have the ability to map one path to multiple routes.

A visual diagram of a basic routing setup.
When a request is made through your browser or mobile device, the routing is used to determine the active controller, and then you receive the appropriate response.
So why the change in Drupal 8? The hook menu was far too complex to fully comprehend what it was doing at any given point, and it was extremely challenging to perform advanced tasks using it.
Without delving into code specifics, in Drupal 8 we’re able to have a function inside a method, inside a class, rather than in one file (because everything in Drupal 8 exists in classes and methods). This means simpler routing while still maintaining functionality.
In Drupal 7, a hook menu would like something like this:
With Drupal 8, we have cleaner code and a simpler system with routing:
For those who were unable to attend, check out our slides below for more on the topic.
In addition to presenting, I also had the chance to work on some Drupal 8 issues in Forum One’s Coder Lounge. It was a valuable opportunity to mentor local developers, meet other Drupal enthusiasts, and even reconnect with some friends I’d met at last month’s Jersey Shore code sprint who made the trek to CapitalCamp to take part in our Drupal core sprints. As Drupal 8 inches closer to beta release, I’m proud to be part of a team that is actively contributing to the Drupal community.
2bits: High Performance Drupal with Apache MPM Worker Threaded Server and PHP-FPM
Stanford Web Services Blog: Troubleshooting the Field Group 7.x-1.4 Update
In July, 2014, the Field Group module was updated from 7.x-1.1 to 7.x-1.1 on Stanford Sites. This update has the potential to cause issues with CSS, as certain types of markup were removed from the HTML output of the page.
BackgroundThe Field Group module allows site builders to group fields together on the back-end edit form of entities (e.g., nodes, BEANs), and on the front-end display of those entities. The latter is what was affected.
Drupalize.Me: Drupalize.Me Free Icon Package
Are you finding yourself searching for some new icons to use on your latest project? Drupalize.Me loves helping out the Drupal community, and people in general, so for this post I thought it would be fitting to provide you with a carefully designed free icon set.
Acquia: The Last-Ditch Fix - Programmatically changing a Drupal 7 view
Originally posted on Yellow Pencil's blog. Follow @kimbeaudin on Twitter
precessionmedia: How To Create A Custom Rules Action
This post should give a quick example on how to write the code to create your own custom actions for Drupals' Rules module. Writing your own plugins for rules (events, conditions or actions) can give you enormous benefits later, when you start to reuse them throughout the site or even port them on other Drupal sites.
We will be creating an action, which will provide a hashed string. In order to create this string we need to pass some parameters to php's hash function like a source string, a list with possible algorithms to choose and an output length. These parameters will be configurable through Rules' backend. So let's dive in.
First you need to create a basic custom module in your Drupal installation. The one I have in my custom environment is called "my_module".
You don't need anything special in your "my_module.info" or "my_module.module" files, but there has to be a file called "my_module.rules.inc", which will hold the code for your rules' action. Create it and add following code to it:
<?php /** * Implement hook_rules_action_info(). */ function my_module_rules_action_info() { return array( 'my_module_rules_action_create_hashed_string' => array( 'label' => t('Create hashed string'), 'group' => t('Custom'), 'parameter' => array( 'string' => array( 'type' => 'text', 'label' => t('String to be hashed'), 'description' => t('Enter a value for a string that will be hashed using the md5 hash-algorithm.'), ), 'length' => array( 'type' => 'integer', 'label' => t('The length of the returned string'), 'description' => t('Enter a number for the length of the hashed string that will be created.'), ), 'algorithm' => array( 'type' => 'text', 'label' => t('Algorithm'), 'description' => t('Select a hash algorithm.'), 'options list' => 'my_module_algorithm_options', 'restriction' => 'input', ), ), 'provides' => array( 'hashed_string' => array( 'type' => 'text', 'label' => t('Hashed string'), ), ), ), ); } // A helper function to provide us with a list of algorithms function my_module_algorithm_options() { $bundles = array(); $bundles['md4'] = t('md4'); $bundles['md5'] = t('md5'); return $bundles; } // This callback creates the hashed string by using the parameters provided through rules' UI function my_module_rules_action_create_hashed_string($string, $length, $algorithm) { if ($length <= 0) { // For anything below or equal zero lets return the default value. $string = hash('md5', $string); } else { $string = substr(hash($algorithm, $string), 0, $length); } return array( 'hashed_string' => $string, ); }Here we implement initially "hook_rules_action_info" and add our own action to it. Our action is an associative array keyed with the name of the callback that will return our value (in this case a hashed string). Inside it we give our action a label and put it in a group ("Custom"). The next part of this array is an associative array itself, keyed with "parameter". Inside it we describe our 3 parameters, which will be passed to the action callback. Note that the "algorithm" parameter has an entry with the key "options list" which points to a helper function ("my_module_algorithm_options") to keep the code more lean.
The last part of the array is another associative array keyed with "provides". This key tells Rules what the machine name of the provided variable is, among with giving it some additional data like type or label. You can use this provided variable in latter actions of your rule now!
The last part of the code is the action callback. Only thing to note here is that we return the whole string returned by the hash-function, when the value of $length is below or equal to 0.
Clear your cache in order for Drupal to register the code you added and the new action should appear now:
In order to see it's working I've added a "Show a message on the site"-action which shows the provided hashed string when we're looking at a node page:
That's it with this simple example! Please leave a comment if there is anything more that comes to mind. Thanks!
By dimitar on 05.08.2014
Share this:



Janez Urevc: Progress of Entity embed module in GSoC 2014
If you want to try the module and/or contribute please visit the project page. You are also invited to check original post on groups.drupal.org.
Cheppers blog: 7 +1 steps to plan a successful Drupal website

According to our experience the most usual approach for clients with web development needs is to contact multiple agencies with more or less vague ideas - asking for quotes, and then selecting a choice based on price. This approach is doomed to fail for two reasons:
- Without a precise specification of requirements, agencies will have to base their quotes entirely on guesses.
- The client is missing out on the value that the agency could have added if they were involved in the discovery and planning as well.
Károly Négyesi: Drupal 8 progress from my / MongoDB perspective: update #28
The standard mechanism for backend-aware service overrides is in and these services are already tagged. We have agreed on how to transfer data from one backend to another and my sandbox contains the first getTransferIterator implementation for config with more to follow. There's a small amendment in the works that makes the old backend available too.
More field renames are in the works. That's why I am not focusing on the MongoDB drivers just yet. Let's wait for beta. The drivers in a state where we can do some meaningful testing (which lead to making sure tests are modifiable before) and so we can make sure everything will work for us but writing the entity drivers themselves at this point is a waste of time -- let's wait for a (more) stable API. The transfer work started because MongoDB needed to solve taking over config storage and while we had it solved, there is really nothing MongoDB specific so I am pushing for core inclusion.
I didn't mention config devel in this series -- although I did previously on this blog -- I firmly believe this will be used widely and lead to a more joyous CMI experience. Reminder: everyone using CMI is what makes the Drupal on MongoDB only feasible in the first place.
Drupal core announcements: Drupal Core Updates for Aug 4, 2014
It's been an exciting two weeks as Twig Autoescaping was turned on by default, the menu links system was completely revamped, entity caching was finally added to core, and we switched Drupal 8 version numbers to Semantic Versioning!
The switch to semantic versioning means that if you have a clone of Drupal 8 core, you shouldn't patch the 8.x branch anymore: use 8.0.x instead. To switch branches, simply run git fetch origin && git checkout -t origin/8.0.x. See jhodgdon's announcement to the Core group for more information.
The valiant efforts of the 15-person team at the Drupal 8 Code Sprint at the Jersey Shore saw 30 issues move forward, 12 of which have already been committed. For more information, read this recap of the event by Kalpana Goel of Forum One.
Finally, thank you to all the contributors who helped us fix 378 Drupal 8 issues in July, 101 more than in June! The fast turnaround in the RTBC queue from our awesome core maintainers has been really motivating — as of right now the RTBC queue is totally empty, meaning that every RTBC issue has either received committer feedback or been committed. (Also noteworthy, Alex Pott of Chapter Three committed a remarkable 70% of July's many commits. Wow!)
Where's Drupal 8 at in terms of release?In the past week, we've fixed 9 critical issues and 22 major issues, and opened 4 criticals and 35 majors. That puts us overall at 104 release-blocking critical issues and 656 major issues.
Only 3 of 173 beta blockers remain before we can release a Drupal 8 beta. Drupal 8will soon be in beta, so now is the time to take a close look at the remaining critical issues and beta deadline issues. In each issue, help clarify:
- If it's critical or major, why?
- What would be the implications of not fixing the issue?
- What would be the implications of fixing the issue between betas? (Code changed for modules, upgrade path, etc.)
- What would be the implications of fixing the issue after the first release candidate?
- What is the next step to make progress? What are the remaining tasks?
Each week, we check with core maintainers and contributors for the "extra critical" criticals that are blocking other work. These issues are often tough problems with a long history. If you're familiar with the problem-space of one of these issues and have the time to dig in, help drive it forward by reviewing, improving, and testing its patch, and by making sure the issue's summary is up to date and any API changes are documented with a draft change record, we could use your help!
- Issue #1934152: Figure out if we want global config overrides to stick (settings.php overrides don't work on all pages) aims to determine if it would be more secure/sane to apply global configuration overrides hard-coded into settings.php even when they wouldn't normally (for example, when editing/previewing a the configuration of a view in the Views UI, where request/URL don't apply because they're intended for the edit page, not the view itself), or whether it would be better to provide a 2-tiered override system (one for global overrides and one for "soft" request/URL overrides).
- Issue #2313159: [meta] Make multilingual views work is a collection of problems related to making multi-lingual views in Drupal 8. A number of the sub-issues are "Major", meaning they have significant repercussions but do not render the whole system unusable.
- Issue #2189661: Replace $form_state['redirect_route'] with setRedirect() aims to make the Form API more consistent with the rest of core, but the patch is out-of-date and needs to be re-rolled.
- Pick a critical issue or beta deadline issue, take the time to thoroughly read the issue (including doing some background reading if necessary to understand the problem space), and then update the issue summary for the issue. Include a summary of the current status and remaining tasks for the issue, and identify any API changes the issue would introduce. Consider whether the change would require a change record or updates to existing change records. Consider what the implications of not resolving the issue would be, or of resolving it after the first beta or after release.
- We also need help writing help text for core modules like Entity, Contextual Links, Field UI, Image, Taxonomy and Toolbar. This is an easy way to learn the Drupal Core contribution process and start contributing to Drupal Core.
- Help brainstorm how to improve core's Contact module for Drupal 8.1 and beyond.
As always, if you're new to contributing to core, check out Core contribution mentoring hours. Twice per week, you can log into IRC and helpful Drupal core mentors will get you set up with answers to any of your questions, plus provide some useful issues to work on.
You can also help by sponsoring Drupal core development.
Notable CommitsThe best of git log --since "2014-07-16" --pretty=oneline (180 commits in total):
- Issue 1825952 by Fabianx, joelpittet, bdragon, heddn, chx, xjm, pwolanin, mikey_p, ti2m, bfr, dags, cilefen, scor, mgifford: Turn on twig autoescape by default
Now, every string printed from a twig template (i.e.: between {{ and }}) is automatically run through String::checkPlain(). This makes it hard for themers and module developers to accidentally introduce XSS attack vectors in their code, which is a big win for security.
If you notice a double-escaping issue, please update Issue #2297711: [meta] Fix double-escaping due to Twig autoescape.
A follow-up issue was also committed: Issue #2289999 by dawehner, Cottser | Fabianx: Add an easy way to create HTML on the fly without having to create a theme function / template.. This makes it easier to generate tiny chunks of HTML where full Twig files would not be useful. - Issue 2256521 by pwolanin, dawehner, Wim Leers, effulgentsia, joelpittet, larowlan, xjm, YesCT, kgoel, victoru, berdir, likin, plach, alexpott: [META] New plan, Phase 2: Implement menu links as plugins, including static admin links and views, and custom links with menu_link_content entity, all managed via menu_ui module.
This critical beta-blocker completely revamped the menu link system on the back-end (the UI for managing menus and menu links remains largely the same). It added a common interface for menu links, to hide implementation details and let different storage methods work together in the same menu tree, condensed the crufty, confusing code that loads and renders menu trees down to just three methods, decoupled breadcrumbs and menu links, and broke down the code into multiple services to allow different behavior to be customized with a minimal amount of code. - Issue 597236 by Berdir, catch, msonnabaum, Xano, Wim Leers, jhedstrom, amateescu, corvus_ch, swentel, moshe weitzman, Gábor Hojtsy, riccardoR, killes@www.drop.org, et al: Add entity caching to core.
This issue, which has been around in various forms for about 10 years, increases overall peformance by caching entities so they don't have to be rebuilt every page request. Initial performance testing showed a performance increase of about 15%, although this varies based on the number of loaded entities. - Issue 1986418 by tompagabor, LewisNyman, idflood, jamesquinton, lauriii, emma.maria, danmuzyka, rteijeiro, scronide, frankbaele, Coornail, ekl1773, oresh, philipz | Bojhan: Update textfield & textarea style.
- Issue 733054 by jhodgdon, mkalkbrenner, amitgoyal, ndewhurst: Fixed Watchdog logging of all searches is performance hit; need ability to turn it off.
- Issue 1288442 by jhodgdon | Wolfflow: Added search index status to the Status Report page.
- Issue 2062043 by eelkeblok, longwave, rhm50, InternetDevels, alvar0hurtad0, Xano: Replace user_access() calls with $account->hasPermission() in core files.
- Issue 2293773 by Gábor Hojtsy, alexpott, effulgentsia, penyaskito, hussainweb: Fixed Field allowed values use dots in key names - not allowed in config.
- Issue 2247049 by sqndr, herom, LewisNyman: Redesign password strength indicator so it's less fragile.
- Issue 2225353 by tim.plunkett: Convert $form_state to an object and provide methods like setError().
You can also always check the Change records for Drupal core for the full list of Drupal 8 API changes from Drupal 7.
Drupal 8 Around the InterwebsIf you want to keep up with the changes in Drupal 8, but you'd rather absorb yourself in articles than dig through diffs, here are some notable blog posts to read:
- Lizzie Hodgson explains user personas, why they're important, and how Deeson Online's content strategists came up with and contributed personas to the Drupal 8 landing page on Drupal.org based on Dries' keynote in Prague.
- Virginia Durikova of Mogdesign discusses the importance of the #d8rules Drupalfund project and analyzes why it was so successful.
- Kim Pepper of PreviousNext wrote a tutorial on how to use Drupal 8 Condition Plugins API.
- Thomas Seidl wrote a tutorial on how to create your own Drupal 8 service.
- Keenan Holloway of Forum One shares a presentation on how to get set up for Drupal 8 development.
- Pedro Rocha of SingleView blogged about his experience using the popular Bootstrap framework to theme a Drupal 8 site.
- Kyle Hofmeyer of Drupalize.Me lists some Drupal 7 modules that provide Drupal 8 features.
- Alex Pott of Chapter Three writes about reviewing Drupal 8 patches with PHPStorm.
August will have many events for you to meet other Drupal contributors and collaborate on the issues you're passionate about! Some notable ones are:
- Aug 7-10: Twin Cities DrupalCamp in Minnesota, USA will have a sprint room for all four days and has a sprint sign-up. @TCDrupal
- Aug 7-10: Drupalaton at Lake Balaton, Hungary has Drupal 8 sessions and sprints. @drupalaton
- Aug 9: Vacation Code Sprint in Wrocław, Poland will be meeting to work together on Drupal 8 at the RatioWeb offices.
- Aug 16-17: Drupal Sprint Weekend in London, England will have new-contributor, core and contrib sprints.
- Aug 22: DrupalGov in Canberra, Australia features everything relating to Drupal and the Australian Government, including Drupal 8 sessions.
- Aug 23: Drupal 8 core sprint in Helsinki, Finland will focus on Drupal.fi and Drupal 8 Core.
Do you follow Drupal Planet with devotion, or keep a close eye on the Drupal event calendar, or git pull origin 8.0.x every morning without fail before your coffee? We're looking for more contributors to help compile these posts. You could either take a few hours once every six weeks or so to put together a whole post, or help with one section more regularly. Contact xjm if you'd like to help communicate all the interesting happenings in Drupal 8!
Friendly Machine: Headless Drupal? It Just Might Be a Bigger Deal than Twig
If you're a frontend developer or designer that has grumbled about the challenges of Drupal theming, you no doubt applauded the announcement that the Twig template framework was being added to Drupal 8.
It's a big upgrade, no question. If you're like me, however, you may prefer a completely custom frontend crafted out of HTML, CSS and JavaScript. You may have looked at the cool stuff AngularJS or Backbone is capable of and wondered how you could bridge the gap with Drupal to enjoy that sort of freedom.
Fortunately, there are some folks that are already doing exactly that and sharing the results of their work. It's something called "headless Drupal" and it's an approach that uses Drupal as a backend content repository and REST server.
A REST server makes it possible for other applications to read and update data. The typical case is that Drupal is used to store and manage content and it then provides that data to your app built with Angular, Backbone, Ember, or whatever. If that's not entirely clear, don't worry. The links below will help sort it out.
Headless Drupal ResourcesHeadless Drupal Manifesto - This is great place to start. It succinctly answers the question of why anyone would want to do this sort of thing.
Headless Drupal Group - A group on Drupal.org devoted to sharing ideas, discussion and experiments around the topic of headless Drupal.
Build a Drupal-free theme with 8's REST API and JavaScript - A presentation from DrupalCon Austin on building an AngularJS site that uses Drupal for the backend.
Headless Drupal, One form at a time - This is a great post from Amitai Burstein that demonstrates some of what this approach has to offer for the creation of frontend user interfaces.
Headless Drupal - Inline edit - Another good one from Amitai.
Here’s Drupal - Tonight on the Tonight Show with Jimmy Fallon - A case study of headless Drupal in action on a very high profile site.
If you know of some other resources, please share them in the comments below. I'd love to check them out.
Nikro: Moldcamp 2014 - a late review

I know, it's been a while since the event took place (17th-18th of May), I was pretty busy and had a lot of stuff to do meanwhile, so I finally found a couple of hours to make a small review.
Tags:Microserve: Coding in the Cloud

An old timer like myself couldn't help but be filled with trepidation when it was revealed I'd be acting as the company guinea pig to give cloud based development a viability road test on my latest project.
No matter how cool I played it, the momentary panic at the thought of working without the tried and tested 'REAL' software apps installed on my macbook, must have been visible on my face.
You'll have to pry my favourite text editor out of my cold dead hands!I've been used to the same comfy workflow for the last few years.
A local environment comprising of the same trusty text editor/IDE, a LAMP stack running on MAMP and code versioning using GIT. There was just something about 'cloud based coding' that didn't seem to ring true.
Pause For ThoughtThen when i really thought about it, I began to examine just how much of my professional and personal life had already drifted into the cloud and in most cases for the better.
Thanks to Google, Dropbox and Apple amongst others, my email, calendars, notepads, files, photos, videos and documents have for the most part been in the cloud for ages. Do I miss opening up MS Word to write a letter? No.
Then I thought about how much easier life as a Drupal developer had become since adopting tools like Drush, Git and more recently, the Pantheon platform. (in essence 'cloud' based tools themselves.) So, thinking about it, cutting the last few remaining ties to local, machine specific workflow seemed less of an imposition and more like the next natural step in the evolution of web development.
Where to start?After a bit of googling 'cloud based IDE', you'll see there's already quite a few choices. Most seem to follow a similar basic subscription paradigm (one or a number of free public projects and a paid for plan for private/production projects.)
In most cases they provide a fully functioning text/code editor with text highlighting support, basic options for uploading, renaming and deleting files, a cloud based LAMP stack, some kind of terminal or command line and a selection of optional 'plugin' tools.
RoadtestI've been testing Codio: https://codio.com/
The site I've been working on is a Drupal 7 site that arrived with us, half built.
Codio handled the import of the existing database and files without much hassle and pulled in the code base via Git.
I was able to install drush as a Codio ‘part' (plugin) and was installing essential modules via Codio's command line terminal in no time.
There's a nice file tree in the left pane and the editor itself is a real pleasure to use.
I was impressed to find text highlighting support for SASS/LESS out of the box and it had decent poke at autocomplete of attributes.
There's a handy tab in the main menu which opens your project 'box' in a new tab or window and on a decent sized screen, it's pretty easy to get a nice comfortable layout with your code in one window and the running site in another.
One of the best things about Codio is that all changes to code are instant, with no saving or incremental Git commits needed. You only need to refresh your site to see it update.
Once you've done a tranche of work you're happy with, you can commit as you normally would, either using the in-built terminal or the GUI menus.
ConclusionBy the end of day one I had been totally sold. The feeling of freedom was, well.. liberating. The next day I had to set up a new mac to use at work, and usually this process eats up half a day of downloading latest versions of software, configuring, chasing licences etc. In this case I literally just turned on the new laptop, installed my browser of choice, logged into Codio and I was away!
Of course, with everything, there are some niggles. The most obvious downside to cloud based development is, that when your connection is spotty or you suffer an outage, you're immediately incapacitated. A few days in and the Codio servers themselves had a few hours of intermediate drop outs. You can imagine how frustrating this might be if you were riding up close to a deadline.
But on the whole the good far outweighed the bad and I'm sure that service and reliability is only going to improve as web based coding becomes the norm... Which i'm now convinced it will before too long.
Pros and Cons of Web Based Development Pros- Code anywhere that has a connection
- Collaborate more easily with other developers
- No local apps needed other than a browser
- Platform agnostic (Makes things like Chromebooks slightly more viable as a stripped down development machine)
- Less chance of incompatible files being shared between collaborators
- Fewer problems for code team Sysadmins.
- Reliant on reliable internet connection and host server
- Basic file, folder housekeeping can be a bit laborious.
*I should point out that my experiences are with Codio IDE, but there are many other alternatives available. Cloud 9 seems to be proving very popular for instance.
You can find a rundown of some of the most popular here:
http://www.hongkiat.com/blog/cloud-ide-developers/
Drupal core announcements: Fixing double-escape bugs
Since Twig autoescape is on, there are double escape bugs. If you can fix them by refactoring code into Twig templates, that's great. If not then wait for an easy way to create HTML on the fly without having to create a theme function / template. It's already RTBC, it's a matter of days to find its way in. Regarding SafeMarkup::set calls, there is an issue to refactor and remove the ones we needed to add to get tests pass and so adding more is not acceptable (the reason for writing this announcement are the quick fix issues I need to whack trying to fix double escape by adding more of these).
Károly Négyesi: OOP developer experience: snap to grid
After writing my first Drupal 8 contrib module I have a new appreciation. Let me try to explain. It is certainly possible to write a letter on an empty sheet of paper but achieving tidy results is quite a lot easier if it's a lined sheet. In Drupal 7, the code flow in general is calling a function, getting an array, manipulating the array and passing it to another function. Pretty much anything goes. In Drupal 8, you are handed an object and the object has methods. Instead of trying to figure out which possible function are you continuing with, it is one of the methods. When passing on data, the receiving method (hopefully) has a helpful type hint which tells you what to pass in. Often even the argument name will be helpful too.
It's exactly like having a lined paper: you still need to know how to produce legible handwriting but at least you have a guide. Another apt metaphor is lining up shapes in a drawing program. Yes, it's possible by hand and there's more freedom doing it but for most it's easier to just use the snap to grid feature.
This blog post was inspired by ConfigDevelAutoImportSubscriber having a $this->configManager object. That I need a config manager I have copied from a core file doing similar things. So, I wanted to create an entity -- I already know doing that requires calling the create method of the relevant storage controller. But how do you get one? Well, ConfigManagerInterface only has 10 methods and only 1 looks even remotely relevant: getEntityManager. So now I have a EntityManagerInterface object. Look, a getStorage method.
<?php
$entity_type_id = $this->configManager->getEntityTypeIdByName($config_name);
$entity_storage = $this->configManager->getEntityManager()->getStorage($entity_type_id);
$entity = $entity_storage->create($data);
?>
We could call this "autocomplete driven development" cos the autocomplete in your IDE pretty much drives you forward. Oh yeah: trying to develope D8 without an IDE is not something I'd recommend.