Software
Aten Design Group: Modularizing JavaScript
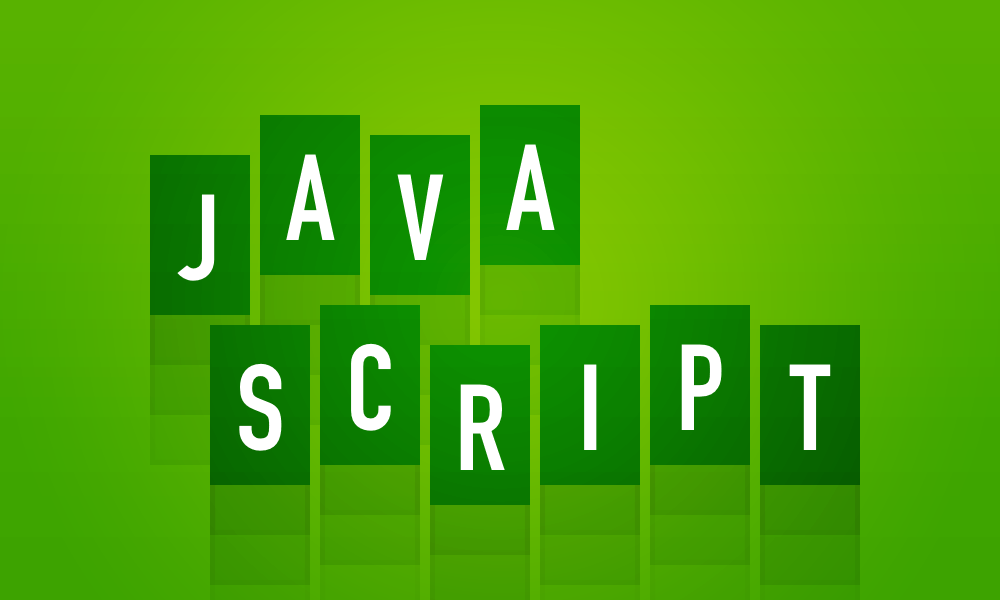
I came to Drupal from a JavaScript background and was happy to see a module system in place. Have you ever wished you could write your JavaScript modularly just like your PHP in order to take advantage of testing, better documentation and the Do One Thing Well philosophy? Well I have the solution for you: Browserify and NPM!
Getting StartedIn order to take advantage of JavaScript modularity you will need node and npm which can be installed together using one of these methods.
Creating a JavaScript ModuleJavaScript modules are similar to the PHP include system in that they allow the developer to separate their code across multiple files. The key difference is that JavaScript uses the syntax require to pull the exported part of a JavaScript file into the current file's variable of choice. Let's take a look.
Simply create a JavaScript file, do whatever you’re trying to do and assign the resulting value (usually a class or object) to module.exports.
// src/lib/MyClass.js module.exports = function MyClass(opts) {} MyClass.prototype.magic = function() { console.log(‘magic’); }You can now use MyClass.js from other JavaScript files like so:
// src/index.js var MyClass = require(‘./lib/MyClass’); var classy = new MyClass(); classy.magic(); // ‘magic’ NPMPHP developers may be familiar with Composer. NPM is the Composer of the JavaScript world. Both allow you to manage the dependencies of your project.
Before creating a custom module it's a great idea to see if it has already been written. npmjs.org is the place to look. When you find a module you’d like to include in your project, retrieve it and add it as a dependency with npm. For example, to install lodash:
npm install lodash --saveThe save flag adds this dependency to the package.json file. It might help to think of this as the JavaScript version of composer.json. All the dependencies for a project can be installed by running npm install within the directory containing package.json or its sub-directories.
Requiring modules from NPM is done just like local modules but you don’t have to provide the path, just use the module name. require will look in the node_modules folder automatically.
var _ = require(‘lodash’);Read more about NPM and package.json on the NPM website.
The Browserify commandBrowserify reads your JavaScript files and resolves their module.exports and require's.
Your main JavaScript file should be a compilation of its dependencies resulting in a useful piece of functionality for your website. To compile your multiple files into one that is browser ready we will use the browserify CLI.
First install it globally with npm. Globally installed modules can be accessed from anywhere on your machine.
npm install -g browserifyThen tell Browserify where your main JavaScript file is and redirect the output to where you want your compiled, browser-ready JS to be.
browserify src/index.js > build/myModule.jsbuild/myModule.js is now browser ready! Now you can add it to your website.
Come see me at BADCampThe above workflow is a great way to maintain custom Drupal modules with a lot of JavaScript. For more detailed information about using this workflow with Drupal — such as global dependencies, handling jQuery, task automation and testing — come check out my session at this year’s BADCamp!
Open Source Training: Node Gallery: The Easiest Drupal Photo Gallery
We have several different Drupal photo gallery tutorials on this blog. The most popular are Views Photo Galleries for Drupal 7 and Node Gallery for Drupal 6.
However, although Views is powerful, it is too complex for many Drupal users and Node Gallery remains popular. So here's an update that shows how to use Node Gallery in Drupal 7.
Node Gallery is still the easiest way to build a photo gallery in Drupal. It requires very little set-up and almost no configuration.
Sooper Drupal Themes: Introducing SooperThemes.com version 5
Welcome to the fifth redesign of sooperthemes.com!
The fifth major update to sooperthemes.com is the biggest update ever. It's a completely new site, unlike the previous sites which were built on top of the original alldrupalthemes.com (2007!)!
The new site comes with a big shift in focus. With Drupal 7 sites becoming more complex, and the upcoming Drupal 8 introducing new power and flexibility, but also complexity, we work hard to offer tools, services and products that allow everyone to profit form Drupal's flexibility without having to suffer the steep learning curve (alone). In addition to selling Drupal themes with turn-key demo sites we now offer more services:
- Custom built ticket system on sooperthemes.com, to work alongside e-mail support
- Design to Drupal service, for affordable and top quality Drupal themes based on your own design
- Enterprise Drupal services, including art direction, design, theming, data driven design and consulting.
- No more one-off theme sales, total focus on club membership and long term support
Our new tools are the best part, read on to learn about our major plans to support Drupal for small/medium businesses.
Drupal Developers will Love these New Tools: A new Distribution: Drupal CMS PowerstartDrupal's awesome architecture is what makes it such an awesome alternative to competing CMS like Wordpress and Joomla, but setting up shop with Drupal is described by many as painful and too complicated. Here, Drupal's power in flexibility is also it's weakness. It takes too much time to install basic features like WYSIWYG with media management: you need to find modules and set up complicated configurations, sometimes needing patches or development versions of modules, external libraries, etc.
To solve this problem, we created a Drupal CMS Distribution, targeted to small business sites and aiming to make life easier for developers and shops who create small sites.
Dries has mentioned in several keynotes that Distributions are the key to make Drupal more accessible and therefore the Drupal ecosystem more succesful. We too believe distributions are the solution but we don't like how they are currently made and how they are managed by drupal.org. Therefore we have created a new interface: A user-friendly interface to our distribution that lets you pick features, so that you don't get a bulky distro with everything in it, and the kitchen sink.
Check out the custom build interface to Drupal CMS Powerstart:
Twitter Bootstrap Fans will love what we did with Bootstrap 3In order to create a truly responsive distribution, we developed and refined a lot Bootstrap 3 Drupal-integration. Responsive grid control from Views, content organisation with Bootstrap shortcodes: https://www.drupal.org/project/bs_shortcodes and all our features come with default layouts that regardless of whether you use a Bootstrap theme.
Development of Drupal CMS Powerstart is sponsored by sooperthemes.com and if you want to support future development of this new platform, please help beta testing and/or consider becoming a member of our premium themes club, you can see our "premium themed" version of CMS Powerstart here: http://glazed-demo.sooperthemes.com/ - more Drupal themes.
Tags sooperthemes drupal 7 drupal 8 distributions cms planet drupal planet cms powerstart Drupal 7.xERPAL: Drupal design patterns – do we need them?
After six years of working with Drupal, I’ve seen many successful Drupal projects. I’ve also felt how hard it is to become an experienced Drupal developer. When I asked myself why some Drupal projects succeed while others don’t, I came to this conclusion: the projects that integrate with Drupal core and the contrib ecosystem – i.e. the ones that use "best practice" deployment workflows and try to use configuration instead of custom code – keep their flexibility, stay maintainable and avoid "niche know-how". This then ensures that the project can be continued even if the team changes. Of course, there are lots of other non-technical factors that make projects successful but we’re focusing here on the technical ones. The problem is that there’s no central repository of "best practices": almost all developers or Drupal shops have their own.
This brings me to the point of considering some Drupal design patterns. Whereas in object-oriented programming, developers have patterns for their object models to solve specific problems that appear again and again, we haven’t yet established any in Drupal. But if we look at the configuration layer of Drupal as a "programming language", we could describe some patterns or best practices that would help others with problems that have already been solved hundreds of times before. This would have the same benefits as design patterns in other programming languages. Solutions for equivalent problems / requirements are always designed in a similar way. For any given workflow we could always use the same rules, so everybody would know where to go to change some behavior. The result would be that modules wouldn’t implement their own logic in code but provide actions, events and conditions, and some default rules. People still wouldn’t find a Drupal image gallery module but they would know how to build one according to some patterns. Of course we’d have a longer dependency chain for some modules, but is this really a problem if we’re depending on standard modules?
When to choose which module – would patterns work for this?
With more than 8000 modules available at Drupal.org, new Drupal users may find it hard to get started. What usually happens is that they enable lots of modules to test them; if the module works for their requirements, it remains enabled. If the user is a technical person, maybe even a developer, he/she will most likely use the Drupal API to extend the Drupal app.
But wouldn't it be great if we had a list of modules that were "state of the art" for best practices such as using rules for workflows and business logic, ECKs and fields for data structure modelling, views for lists and data queries, restWS and WSclient for communication with other applications, entity view modes in combination with panels for layouting, and features for the deployment. Considering the above, I’ve created the Drupal application stack poster to collect all our modules – along with their specific use cases –and to share this overview with the community. Shouldn't we always have a "Drupal-relevant set" of modules in mind when it comes to the modelling process of our Drupal app? This would keep Drupal apps sustainable and flexible.
Dos and don’ts
There are also some examples of what not to do. In a few projects that had crashed before they came to us to be rebuilt, it became clear that the previous developers had exactly the problems listed above. They had no guide to help them figure out when to use the API and when to choose which module, which further led to code and architectures that nobody but the developer could maintain. After the first release, the projects weren’t extendible, as there was no deployment process established. Wouldn't it help to collect these examples as well, to help people avoid them and get on the right path with Drupal? With a list of positive and negative patterns, we could give users some objective criteria for rating the implementation of their Drupal project.
Distributions – start from a higher level
Distributions built on such best practices as Drupal commerce kickstart or ERPAL Platform provide a good starting point for new Drupal developers, since they can see how experienced Drupalistas created the distros and which modules they used. Distros can be showcases for Drupal design patterns or a kick-start for vertical use cases like e-commerce sites or business applications.
If we want the Drupal community to grow, we should help others find the right starting point – not just any old one that could lead them off in the wrong direction: while they might see some quick results, if it wasn’t the right approach, they would only realize that after days of work. Once too much time has elapsed, with the money already spent and the deadline looming ever closer, nobody will ever refactor the Drupal application to improve its structure.
With Drupal design patterns, we’d be able to offer standard solutions to often-complex problems. What are your thoughts about Drupal design patterns? Would this bring us another step closer to making Drupal into a world-leading web application framework?
Drupalize.Me: Free Halloween Icon Set
With Halloween just around the corner, I thought it might be fun to hand out some tasty treats. Don't worry, you're not getting fruit or pennies. It's something much more fun! I designed a custom Halloween icon set, which is free to download and use however you want. With Drupalize.Me's scary good Drupal training, it's fitting that these icons can spook, sweeten, or surprise your next project.
Clemens Tolboom: Which route belongs to which path fragment?







Visualization some complex part of Drupal is helping understand Drupal better. This is about the menu tree using Graph Viz for a static SVG diagram and D3JS for a dynamic version.
KnackForge: Ajax Autocomplete Customization for Textfield in Drupal
Autocomplete is a feature for textfields in Drupal. It provides a dropdown list of matching options from the server. It is implemented through AJAX. To know how to add an autocomplete form element in Drupal visit https://www.drupal.org/node/854216
For customizing autocomplete we need to override the Drupal system file "misc/autocomplete.js". This can be achieved ideally in two ways:
-
Replace the entire "autocomplete.js" with your customised version
function MY_MODULE_js_alter(&$javascript) { $javascript['misc/autocomplete.js']['data'] = drupal_get_path('module', 'MY_MODULE') . '/js/autocomplete.js'; } -
Override "Drupal.ACDB.prototype.search" with a custom behaviour in theme script.js or module js file, as long as it's added after /misc/autocomplete.js it will override it.
Visitors Voice: “Can our site search be like Google?”
Chapter Three: Drupal 8 Administration is Faster, Cheaper and Easier
Drupal 8 is the most fully featured Drupal version ever. Site builders will notice this most immediately when looking at what is available out of the box. Drupal 8 is faster because features that are expected of a modern CMS, like a WYSIWYG editor, are in Core. It's cheaper because you don't have to pay for custom development to change administration listings since they are Views And it's better because there are less "why does this not work" moments including the ability to place the same block in multiple regions. Below are four videos that demonstrate these new Drupal 8 features and a few more.
Create Custom Administration Experiences
KnackForge: To check Caps lock is on/off status in jQuery
Sample HTML code
a-fro.com: Keeping Compiled CSS Out of your Git Repository on Acquia
A couple of months ago, after a harrowing cascade of git merge conflicts involving compiled css, we decided it was time to subscribe to the philosophy that compiled CSS doesn't belong in a git repository.
Mon, 10/27/2014 - 12:13 aaronTimOnWeb.com: How To Force Search API To Reindex a Node / an Entity
By default Search API (Drupal 7) reindexes a node when the node gets updated. But what if you want to reindex a node / an entity on demand or via some other hook i.e. outside of update cycle?
Turned out it is a quite simple exercise. You just need to execute this function call whenever you want to reindex a node / an entity:
Drupal Tags drupal 7, drupal planet Read on about How To Force Search API To Reindex a Node / an EntityKnackForge: Add class to image tags and panel titles
Nowadays twitter bootstrap theme has become famous among Drupal world due to its flexibility for responsive websites. It is very easy to apply responsive css to the web page by adding appropriate bootstrap classes. If you are new to the twitter bootstrap theme, see here http://getbootstrap.com/css/ for more details about classes. Is it easy to add class to drupal site pages ? If yes, what to do to add class to panel title and image tags ? Lets come to the heart of the topic.
Adding class to panel title
Eventhough it is easy to apply responsive css to the web page, when comes to drupal site we need to follow some standard approaches for adding classes. I need to add class to panel title in one of my requirements. By sticking with standard approach for this, I found this hook template_preprocess_panels_pane very useful to add class to panel title. Below code snippet will explain more detail about the usage.
function themename_preprocess_panels_pane(&$variables) { $variables['title_attributes_array']['class'][] = 'your class'; }The above code snippet need to be written in theme's template.php file.
Adding class to img tags
Then I need to add class to img tags to make the image responsive as one of my requirements. Similar to panel, I found this hook template_preprocess_image very useful to achieve this. See the below code snippet to know in detail
Drupalize.Me: Using A Remote Debugger With CasperJS and PhantomJS
Earlier this year fellow Lullabot Juampy wrote about how we've started using CasperJS at Lullabot in order to do regression testing. I haven't had much chance to dig into it until recently, when we decided to implement some CasperJS tests for Drupalize.Me. So far, I'm really enjoying it—except for those occasions where something in my test is simply not working, and I end up spending hours asking CasperJS to take screenshots using console.log(), and trying to figure out what is going on. Fed up with this process I wanted a debugger.
Larry Garfield: On Drupal's Leadership
My DrupalCon Amsterdam Core Conversation on Managing Complexity has generated quite a bit of follow-up discussion. That's good; it's a conversation we as a community really need to be having.
There are a few points, though, that I feel bear clarification and further explanation as I fear the point of the talk has gotten lost in the details.
Before continuing, if you haven't yet I urge you to watch the session video as well as the background resources linked from the session page. This is not a new conversation; it's the latest chapter in a very long-running discussion that is larger than the Drupal project, and it behooves us all to be aware of the history and context around it.
Károly Négyesi: Drupal 8 critical issues office hours Oct 24, 2014
This was our first critical office hours. webflo have forward ported a Views SA (turned out that Twig autoescape made short work of the security hole -- yay! so now it's just a test) and even past the office hours followed up with a patch that now passes. I will monitor the issue further and make sure it gets reviewed and committed. ksenzee started on decoupling cache tags from cache bins -- there's no patch yet, I need to follow up on this one however from our discussion it was clear she was making a lot of progress. I was trying to help penyaskito with the language.settings config is not scalable issue but turned out his problems went away with a fresh install so that issue is now progressing well even without the office hours. So as far as I am concerned, that's two down and one moving (and as a bonus, webflo rerolled fix common HTML escaped render #key values due to Twig autoescape which is major I am not sure why it's not critical). I think critical issues office hours was off to a good start, more people would of course be better. I count 123 critical issues.
IXIS: Strengthening our Relationship with the British Council

We are delighted to be working with the British Council on a new Drupal hosting and infrastructure support project. The British Council are valued clients, and we have worked with them for more than 6 years managing both the global suite of 150 country sites, and the prestigious suite of Drupal teaching and learning sites.
We will be working to to create four individual platforms for hosting key Drupal websites on, moving away from just one main infrastructure, to improve resilience, efficiency and increase availability to the sites which generate more than 35 million page impressions per month and are used by more than 65 million people each year alone.
Mediacurrent: Culture, Code and Karaoke

What is “culture” and why do we take so much time trying to define it? Is it really important or just another buzzword? This past weekend, we were afforded the opportunity to have a company retreat, that went the distance in proving that culture is something that can’t be bought and paid for, it’s something unique to us and our success really does hinge on its influence. Our people, our relationships, our “culture” is what makes Mediacurrent, Mediacurrent.
Acquia: Learning from hackers a week after the Drupal SQL Injection announcement
Since October 15th, hackers have been busy coming up with creative ways to exploit the SQL Injection in Drupal 7 sites revealed by SA-CORE-2014-005. A week has already passed, and attacks are still ongoing. In a previous post, Moshe Weitzman explained how we were able to protect our customers' sites the moment the vulnerability was announced.