Software
S1L: Selling Organic Groups with Drupal Commerce License OG

Selling Organic Groups with Drupal Commerce just got way more powerful. Actually it did so a while ago when Commerce License and Commerce License OG where created.
About 18 months ago I wrote about how you could sell access to Organic Groups with Drupal Commerce with a configuration of fields and Rules.
With Commerce License and Commerce License OG selling access to Organic Groups you have a setup that is as easy to setup than the 'old' field+Rules way (if not easier) and you'll have great new functionality for revoking membership access.
Step by Step instructionsYou can find the step-by-step instruction on how to sell your Organic groups with Drupal Commerce based on Commerce Licenses at https://www.drupal.org/node/2366023. Just follow the 8 easy steps and you'll have it setup in no-time.
How does it work?Basically you'll be selling licenses to your Organic Group (content). These licenses can expire, or be forever. You can configure them the way you see fit. The license determines if a user has access to the Organic Group or not.
Commerce License is a framework for selling access to local or remote resources.
Read more about Commerce Licenses at https://www.drupal.org/node/2039687 under Basic Concepts -> License.
Show meIf you follow the 8 steps in the instruction at https://www.drupal.org/node/2366023 you'll see that you can easily configure the products like this:
and users on the site will be given licenses like this
From Dev to Stable
commerce_license_og module is currently in dev state. It works fine for the most common usecase: users buying access to your site. However make sure it works the way you want it before you decide go 'all in' implementing this on a production site.
Currently there seems to be an issue with granting anonymous users access to Organic Groups (https://www.drupal.org/node/2366155).
Please add your input to https://www.drupal.org/project/issues/commerce_license_og to help developing this module to a stable release.
Category: Drupal Planet Drupal Commerce Drupal Organic GroupsDrupal Association News: Thank You, Drupal Association Supporting Partners
At the Drupal Association, we believe it’s good to pause and be grateful from time to time. So, today, we’d like to express gratitude for our unbelievably fantastic Supporting Partners.
Without contributions from partners like Exove, Duo Consulting, Chapter Three, Lullabot, Chromatic, The Cherry Hill Company, Elevated Third, and Technocrat, we wouldn’t be able to do what we love: serve the Drupal community and help make Drupal great. Our partners are helping us fund improvements to Drupal.org.
Our partners are making several key intiatives for Drupal.org possible, including:
- Better account creation and login
- Organization and user profile improvements
- Responsive redesign of Drupal.org
- Issue workflow and Git improvements
- Make Drupal.org search usable
- Improved tools to find and select projects
- Groups migration to Drupal 7
In addition to major projects, Supporting Partner contributions make the ongoing work to sustain and maintain Drupal.org happen:
- We performed user research to guide our work on new features and improvements;
- We are starting work on a comprehensive content strategy for Drupal.org;
- We’ve bought and installed new server hardware to improve performance and responsiveness;
- We are improving development environments to make development for Drupal.org faster and more efficient;
- We have faster testbot instances for Drupal 8 development and contribution sprints;
- We implemented content delivery networks (CDN) for Drupal.org and downloads;
- We are improving behavior driven development (BDD) tests to ensure Drupal.org deployments are successful and error free, and many more.
This is only a small portion of the many improvements we’ve been able to make with the help of our Supporting Partners. From helping us make Drupal.org better to making it easier than ever to give back, the Drupal Association’s many wonderful Supporting Partners are some of the heroes of the Drupal project.
Drupal Watchdog: RESTfulness and Web Services
One of the most anticipated features in Drupal 8 is the integration of RESTful Web Services in Drupal core. Drupal devs are looking forward to being able to do things with core which they couldn't before, such as:
- Offering their site’s data up in an API for others to use and contribute to;
- Building better user interactions by adding and updating content in place instead of a full page submission;
- Developing iPhone and Android apps that can serve and manage content hosted in a Drupal site.
But what are RESTful Web Services? In this article, I will walk you through the different conceptions of what is RESTful and explain how the new modules in Drupal core address these different concepts.
A Quick History of REST Many developers have become aware of REST due to the rising popularity of APIs. These APIs enable developers to build on top of services such as Twitter and Netflix, and many of these APIs call themselves RESTful. Yet these APIs often work in extremely different ways. This is because there are many definitions of what it means to be RESTful, some more orthodox and others more popular.
The term REST was coined by Roy Fielding, one of the people working on one of the earliest Web standards, HTTP. He coined the term as a description of the general architecture of the Web and systems like it. Since the time he laid out the constraints of a RESTful system in his thesis, some parts have caught hold in developer communities, while others have only found small – but vocal – communities of advocates.
For a good explanation of the different levels of RESTful-ness, see Martin Fowler’s explanation of the Richardson Maturity Model.
What is RESTful?So what are the requirements for RESTfulness?
LightSky: Drupal Press Shouldn't be Bad
This has been an interesting couple of weeks for Drupal, and that platform as a whole has received a lot of press. With the release of Drupal 7.32, a major (I use this term lightly) security vulnerability was corrected. Drupal then announced this week that, despite there being no significant evidence of a large number of sites attacked, any site that wasn't patched within a 7 hours of the patch release should consider itself compromised. Hosts were reporting automated attacks beginning only hours after the patch announcement. The vulnerability was unprecedented for the Drupal community, but really it shows why Drupal is great, and isn't a black mark on Drupal in our eyes.
First lets look at the announcement by the Drupal Security Team this week, where they say that sites were beginning to be attacked within hours of the patch announcement. The biggest thing to take from this announcement is the words Drupal Security Team. Yep, Drupal has one. I did a search this morning using the following criteria "<popular CMS> security team", and I found the results quite interesting. When I added Drupal as the "popular CMS" I got a page full of Drupal Security team information, policies and procedures. For every other CMS I tried, I got nothing about a team of security people, but a lot of information stating that they are secure and if you find a problem here is how to report it. Drupal focuses on security, and the Security Team at Drupal is a prime example of how important this really is to the Drupal community.
The second thing to take away from this is that the patch really notified the world that there was a vulnerability, and there is no way to stop this from happening. We didn't have any mass attacks on Drupal sites prior to this release, and the damage here after the release seems to be primarily related to those who chose not to apply the updates as they were instructed to. This really emphasizes the importance of applying available updates. Sites where the update was applied quickly likely did not experience any negative effects of the vulnerability, and if they did it was very limited. Updates to Drupal are certainly optional, but they are necessary to avoid headaches down the road, and this is proof of exactly why.
So don't be discouraged by all of the bad looking press related to this. I still stand by the idea that Drupal is the most secure platform available, but it is only as secure as you allow it to be. If you aren't applying the updates as they are available, you are likely putting your self at risk to have your site compromised. The big difference I see between Drupal and the other CMS options is that Drupal works diligently to fix module and core vulnerabilities as a habit. Many others aren't as diligent.
For more tips like these, follow us on social media or subscribe for free to our RSS feed and newsletter. You can also contact us directly or request a consultation.Four Kitchens: Testing Drupal with CasperJS
In our last post we used CasperJS to rapidly test the user interface of a website. Now we will build on these skills and add a familiar element into the mix: Drupal. Like any framework, Drupal offers many predictable, standard behaviors which we can take advantage of. Using this predictability, we can easily test many behaviors including logged-in activity such as posting content.
Testing JavaScript DrupalBluespark Labs: Follow the readiness of the top 100 modules for Drupal 8 with our automatically updated tool
With the first Drupal 8 beta having been released at Drupalcon Amsterdam, we thought this would be a good time to a look at the top 100 projects on drupal.org to see just how far along the line the process of preparing for Drupal 8 is. However, given that there's a lot of progress to be made and I don't feel like manually updating a long list of modules, I decided to make a small tool to get the status of these modules and keep the data up to date.
This turned out to be a fun little project, and slightly more involved than I anticipated at first. (Isn't it always the case!) However, at its heart it's a bone-simple Drupal project - one content type for the Drupal projects (and their metadata) we're interested in, and a few views to show them as a table and calculate simple statistics. The work of updating the metadata from drupal.org is handled in 85 lines of code, using hook_cron to add each project to a Queue to be processed. The queue callback borrows code from the update module and simply gets release data, parses it, and updates the metadata on the project nodes. In the end, the most work was doing the research to determine which projects are already in core, and adding notes about where to find D8 upgrade issues and so on.
So, how did it all turn out? Using the current top 100 projects based on the usage statistics on drupal.org, our tool tells us that as of today, out of the 100 most popular projects:
Thanks for reading, and be sure to keep an eye on the status page to see how the most used contrib modules are coming along!
Tags: Drupal PlanetDrupal 8Open Source Training: How to Check Your Drupal Site Security
If you weren't able to update your Drupal site within a few hours on October 15th, you may be worried about your site.
Even under normal conditions, it's almost never possible to prove that a site is 100% safe. But by checking your site, you can either give yourself some additional peace of mind or you can confirm that you were hacked.
PreviousNext: Drupal 7.32 critical update: Our Response
With the Drupal Security team's release of a public service announcement, the infamous security update known as 'SA-005' is back in the news. Even though it's old news, we've been fielding a new round of questions, so we thought we'd try to clear up some of the confusion.
Modules Unraveled: 124 Creating Drupal Configuration in Code Using CINC with Scott Reynen - Modules Unraveled Podcast
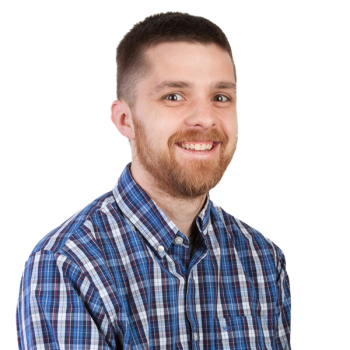
- What is CINC?
- How is it different from Features or Configuration Management?
- Is it something you use on an ongoing basis? Or is it just for the initial site setup?
- What types of configuration can you manage with CINC?
- What if you already have a content type created, and you want to add a field to the content type?
- How does that affect existing content, and new content.
- What about the reverse? Can you remove a field?
- What happens to the data that is already in the database?
- Can you undo configuration that you’ve created with CINC?
- How do you prevent site admins from disabling the module and deleting their content types?
- CINC YAML
- CINC & Features
- CINC & Drupal 8 Config API
- cinc.io
- Sheet2Module
- How do you see CINC working in a headless Drupal setting?
- Create dozens of fields quickly.
- Add a field to a content type after an existing field.
- Update configuration only if it still matches the default settings.
- How do you use this in a dev/staging/production
- Have you noticed any improved feedback, improvements to your workflow while using CINC?
- If people want to jump in and help development or work on new features what should they do?
BlackMesh: Looking at DrupalCon Amsterdam Sprints, Upcoming sprints for you to attend
By:
Tim Erickson, stpaultim, @stpaultim from Triplo
Alina, alimac, @czaroxiejka
Cathy Theys, YesCT, @YesCT from BlackMesh
DrupalCon is a great place to enhance your Drupal skills, learn about the latest modules, and improve your theming techniques. Sure, there are sessions, keynotes, vendor displays, and parties... like trivia night!
But.. there is also the opportunity to look behind the curtain and see how the software really gets made. And, more importantly, to lend your hand in making it. For six days, three both before and after DrupalCon, there are dedicated sprint opportunities where you can hang out with other Drupalistas testing, summarizing issues, writing documentation, working on patches, or generally contributing to the development of Drupal and the Drupal community.
We want to share some details about the DrupalCon Amsterdam Sprints (and pictures to reminisce about the good times) and mention some upcoming sprints that you can hopefully attend.
- Sponsors supporting the sprinters
- Pre-con Extended sprints on Saturday and Sunday (60 Saturday, 100 Sunday, 180 Monday)
- During the con
- Friday Sprint (450 people)
- Post-con Extended sprints on Saturday and Sunday (80 Saturday, 60 Sun)
- Feedback about the sprints
- Upcoming sprints
- Drupal Association, @DrupalAssoc
- Acquia (Large Scale Drupal), @Acquia
- Open8, @open8roger
- Bluehost, @Bluehost
- David Hernandez, @davidnarrabilis
- Wunderkraut, @Wunderkraut
Our sponsors helped us have:
-
Space:
- Co-working space Saturday and Sunday before the con.
- Sprint space at the venue Monday-Thursday.
- Big sprint space Friday.
- Co-working space Saturday and Sunday after the con.
- Food and coffee all of the days.
- Sprint supplies: task cards, stickers, markers, signs, flip charts.
- Mentor thank you dinner.
During the weekend before DrupalCon 60 people gathered on Saturday and 100 on Sunday at The Berlage, a fantastic old castle just blocks from the central train station. On most days the Berlage serves as co-working space. For 48 hours it was home to contributors working together on Drupal core, contrib projects, distributions and Drupal.org itself. Our supportive sponsors supplied lunch and coffee on both days while contributors worked on a number of initiatives: Multilingual, Drupal 8 criticals and beta blocking issues, Headless Drupal and REST, porting contrib projects to Drupal 8, Drupal 8 Frontend United, Search, Drupal.org, Behat (Behavior Driven and javascript/frontend testing), Commerce, Panopoly, Rules, Media, Documentation, Migration, Performance, Modernizing Testbot, and more.
The outside of the Berlage co-working space (castle) with the Drupal
Association banner.
(photo: @gaborhojtsy)
Sprinters sprinting inside the cool looking Berlage.
marthinal, franSeva, estoyausente, YesCT, Ryan Weal
(photo: @gaborhojtsy)
We had lots of rooms for groups to gather at the Berlage.
pwolanin, dawehner, wimleers, Hydra, swentel
(photo: @Schnitzel)
Sutharsan, yched, Berdir
(photo: @Schnitzel)
On Monday sprint attendance grew to 180 sprinters. We moved to the conference venue, Amsterdam RAI. Other pre-conference events taking place included trainings, the Community Summit, and the Business Summit. At this particular DrupalCon there was much excitement about the anticipated beta release of Drupal. Many people did a lot of testing to make sure that the beta would be ready.
Discussing a beta blocker issue they found.
lauriii, sihv, Gábor Hojtsy, lanchez
(photo: @borisbaldinger)
Lots of people sprinting and testing the beta candidate, with support from
experienced core contributors walking around and helping.
tstoeckler, mauzeh
(photo: @borisbaldinger)
Sprinting continued during the conference, Tuesday through Thursday. And, to prepare for Friday's mentored sprint, the core mentoring team scheduled a series of 8 BOFs (‘Birds of a Feather’ or informal sessions). Preparations included mentor orientation, setting up local environments, and reading, updating, and tagging issues in the Drupal issue queue. Mentoring BoFs were open to all conference participants.
Mentor Training
YesCT, sqndr, -, -, lazysoundsystem, neoxavier, Mac_Weber, patrickd,
roderik, jmolivas, marcvangend, -, realityloop, rteijeiro
(photo: stpaultim)
To promote contribution sprints, mentors volunteered at the mentoring booth in the exhibition hall during all three days of DrupalCon. Conference attendees who visited the booth learned about the Friday sprints. Mentors also recruited additional mentors, and encouraged everyone to get involved in contributing to Drupal.
The mentor booth with lots of signage, and welcoming people.
mradcliffe, kgoel
(photo: stpaultim )
At the booth, conference attendees were able to pick up our new contributor role task cards and stickers which outlined some of the various ways that people can contribute to Drupal and provided them with a sticker as recognition for the specific roles that they already play.
Task cards and stickers
(photo: @HornCologne)
In Amsterdam, 450 people showed up to contribute to Drupal on Friday.
(photo: _SteffenR)
People gathered in groups to work on issues together.
-, -, -, -, -
(photo: @peterlozano)
For many people the highlight of the week is the large “mentored” sprint on Friday. 180 of the 450 participated in our First-time sprinter workshop designed to help Drupal users and developers better understand the community, the issue queues, and contribution. The workshop helped people install the tools they would use as contributors. Another 100 were ready to start work right away with our 50 mentors. Throughout the day people from the first-time sprinter workshop transitioned to contributing with other sprinters and mentors. Sprinters and mentors helped people identify issues that had tasks that aligned with their specific skills and experience.
The workshop room.
(photo: stpaultim)
Mentors (in orange shirts): rachel_norfolk, roderik
(photo: stpaultim)
Hand written signs were everywhere!
(photo: stpaultim)
A group picture of some of the mentors.
mradcliffe, Aimee Degnan, alimac, kgoel, rteijero, Deciphered,
emma.maria, mon_franco, patrickd, 8thom, -, lauriii, marcvangend, ceng,
Ryan Weal, YesCT, realityloop, -, lazysoundsystem, roderik, Xano, David
Hernández, -, -, -, -
(photo: @Crell)
Near the end of the day, over 100 sprinters (both beginners and veterans) gathered to watch the work of first time contributors get committed (added) to Drupal core. Angie Byron (webchick) walked the audience through the process of evaluating, testing, and then committing a patch to Drupal core.
Live commit by webchick
webchick, -, -, marcvangend
(photo: Pedro
Lozano)
On Saturday after DrupalCon 80 dedicated contributors moved back to the Berlage to continue the work on Drupal core. 60 people came to contribute on Sunday. During these final days of extended sprints, Drupal beginners and newcomers had the chance to exercise their newly acquired skills while working together with some of the smartest and most experienced Drupal contributors in the world. The value of the skills exchanges and personal relationships that come from working in this kind of environment is cannot be underestimated. While there is an abundance of activity during Friday’s DrupalCon contribution sprints, the atmosphere during extended sprints is a bit more relaxed. Attending the pre and post-con sprints gives sprinters time to dive deep into issues and tie up loose ends. After a number of hallway and after-session conversations, contributors working on specific Drupal 8 initiatives meet to sketch out ideas, use whiteboards or any means of note-taking to make plans for the future.
LoMo, Outi, pfrenssen, lauriii, mortendk, emma.maria, lewisnyman
(photo: stpaultim)
Aimee Degnan, Schnitzel, dixon, -, Xano, alimac, boris, Gábor Hojtsy,
realityloop, YesCT, justafish, eatings, fgm, penyaskito, pcambra,
-
(photo: stpaultim)
-, jthorson, opdavies, drumm, RuthieF, -, -, killes, dasrecht
(photo: stpaultim)
- Sprinting for the First Time - Blog post by AdamEvertsson
- From Rookie to Drupal Core Contributor in One Day - Blog post by @dmsmidt
- DrupalCon Amsterdam, 2014 - Blog post by @valvalg
- “Mentoring at #DrupalCon sprints is the most rewarding and enjoyable part of the week :) <3 @drupalmentoring #DrupalSprint - Original Tweet from @emma_maria88
- “One hour at the #DrupalCon code sprint and I've already submitted my first patch. It is going to be a good week.” - Original Tweet from @skwashd
- Hi, I'm George! I'm your mentor! - Blog post by Thamas (@eccegostudio)
Please contact me to get your DrupalCon Amsterdam sprint related blog added to the list here.
Upcoming sprints- BADCamp (sprint details November 5 - 10 2014)
- Global Sprint Weekend January 17, 18 2015
- DrupalCon Latin America in Bogota (sprint details Feb 8 - 13 2015)
- lots of camps, check druplical.com (The drupal event location visualization tool.)
- Drupal Dev Days April 2015
- DrupalCon North America in Los Angeles (sprint May 9 - 17 2015)
- DrupalCon Europe in Barcelona (sprint Sept 19 - 27 2015)
Plan your travel for the next event so you can sprint with us too!
CorrectionsIf there are corrections, for example of names of people in the pictures, please let me know. -Cathy, @YesCT, or Drupal.org contact form.
DrupalSprintsDrupalConDrupal Planet
Gizra.com: RESTful Discovery - Who knows about your API?
As extremely pedantic developers we take documenting our APIs very seriously. It's not rare to see a good patch rejected in code review just because the PHPdocs weren't clear enough, or a @param wasn't declared properly.
In fact, I often explain to junior devs that the most important part of a function is its signature, and the PHPdocs. The body of the function is just "implementation details". How it communicates its meaning to the person reading it is the vital part.
But where does this whole pedantic mindset got when we open up our web-services?
I would argue that at least 95% of the developers who expose their web-service simply enable RESTws without any modifications. And here's what a developer implementing your web-service will see when visiting /node.json:
Mediacurrent: 10 Things I Wish I Knew About Drupal 2 Years Ago

They say that hindsight is 20/20. With the many advances that have happened in the Drupal community recently, we asked our team "What is the one thing you wish you knew about Drupal two years ago?"
"I wish I knew about the Headless Drupal initiative so I that I could be ahead of the curve as far as the Javascript technologies that it will require." - Chris Doherty
Metal Toad: Seeing Long Term Technology Adoption as Evolution
Much like an evolutionary tree our goal in technology adoption is too continue to move forward and evolve, rather than getting caught in a dead end. In the natural world, becoming bigger can be good but can lead to extinction events should the environment or food source change. Right now we are in a technology Jurassic...
Code Karate: Finding the right brand
If you have been around CodeKarate.com for awhile you have noticed that our branding has been, we
Acquia: Drupal in the Philipines, Own your Own Code & More - Luc Bézier
"Like a lot of people, I did both sides of technology; working on paid, proprietary systems [and open source]. There is a big difference. I can't imagine myself going back to any proprietary system where I have to pay; I can't share the code I am doing with anyone; I have to ask a company about the right tool to use. I love the way that everybody contributes to the same piece of code, trying to make it the best ... and for free!"
The Cherry Hill Company: Islandora Camp Colorado - Bringing Islandora and Drupal closer
From October 13 - 16, 2014, I had the opportunity to go to (and the priviledge to present at) Islandora Camp Colorado (http://islandora.ca/camps/co2014). These were four fairly intensive days, including a last day workshop looking to the future with Fedora Commons 4.x. We had a one day introduction to Islandora, a day of workshops, and a final day of community presentations on how Libraries (and companies that work with Libraries such as ours) are using Islandora. The future looks quite interesting for the relationship between Fedora Commons and Drupal.
- The new version of Islandora allows you to regenerate derivatives on the fly. You can specify which datastreams are derivatives of (what I am calling) parent datastreams. As a result, the new feature allows you to regenerate a derivative through the UI or possibly via Drush, which something the Colorado Alliance is working to have working with the ...
groups.drupal.org frontpage posts: Drupal Camp Ohio
"DrupalCamp Ohio 2014 returns to The Ohio State University's Nationwide and Ohio Farm Bureau 4-H Center, for another two-day camp to build on last year's success, and will include keynote speeches, topical sessions, Beginners Training, Birds of a Feather breakouts, and code sprints."
-- http://drupalcampohio.org/about
Drupal core announcements: This month in Drupal Documentation
This is the monthly update from the Documentation Working Group (DocWG) on what has been going on in Drupal Documentation. Because this is posted in the Core group, comments for this post are disabled, but if you have comments or suggestions, please see the DocWG home page for how to contact us.
Drupalcon Documentation SprintA small group of sprinters gathered on the last day of Drupalcon to work on a couple of Documentation issues. During the sprint the Installation guide was being reviewed for Drupal 8 and there is still work left to process some of that feedback. Furthermore a start was made writing the Theming guide for Drupal 8 (see below for more).
Theming guide for Drupal 8The theming guide for Drupal 8 has been written almost from scratch and is beginning to take shape. It already covers such topics as:
- Defining a theme with an .info.yml file
- Theme folder structure
- Working With Twig Templates
- Adding stylesheets (CSS) to a Drupal 8 theme
- Creating a Drupal 8 sub-theme
- Using Classy as a base theme
- Adding JavaScript to a Drupal 8 Theme
The theming guide still needs a lot of improvement. So if theming is your thing and you have some spare time this month, then please review it. Every little improvement is helpful. Note that you can edit documentation pages on drupal.org directly and that there is no need to leave comments.
CreditsSander Tirez's theming guide, many stackexchange Q&A's and various blog posts proved invaluable input for writing the guide.
Thanks for contributing!Since our last post from October 1 about 280 contributors have made some 1100 Drupal.org documentation page revisions, including 10 people that made 15 or more edits. Thank you batigolix, Pierre.Vriens, SERVANT14, Anthony Pero, camorim, sanchiz, tvn, Pere Orga, LoMo, phenaproxima and all the others and keep up the good work!
Changes to documentation on drupal.org- The formatting of keywords in the sidebar of documentation pages in drupal.org has been improved.
- The display of the original author of documentation pages has been removed. This was done because in many cases the wrong original author was being shown. Now only the most recent revision authors are being shown.
The monthly meeting of the Documentation Working Group is open to anyone who would like to attend. Ping us if you want to join the meeting.
Documentation PrioritiesThe Current documentation priorities page is always a good place to look to figure out what to work on, and has been updated recently.
If you're new to contributing to documentation, these projects may seem a bit overwhelming -- so why not try out a New contributor task to get started?
Drupal Watchdog: Drupal 8 Modules
This article will be more about the patterns you need to use during Drupal 8 development than how to fit the various pieces together.
There’s good reason for this approach: fitting the pieces together has plenty of examples, change records, and whatnot – but many pieces of the puzzle are entirely new to Drupal developers.
The first half of this article provides general PHP information which uses Drupal as an example, but is not Drupal specific. The idea behind this is that the knowledge can be reused well (indeed, this was also a design goal for Drupal 8).
Classes, Objects, InterfacesIn Drupal 7, stdClass was used at a lot of places; ergo, classes (like stdClass) and objects (instances of a class, like node, user, etc.) should be familiar. stdClass is a class without methods, and the properties are not defined ahead of time. In PHP, it’s valid to set any property on an object, even if it’s not defined on its class. So, stdClass worked much like an associated array, except that it used arrows instead of brackets. Another important distinction between arrays and objects is passing them to a function: in PHP5, if a function/method gets an object and then changes the object, it will affect the object everywhere – objects are not copied every time, while arrays are.
Aten Design Group: Modularizing JavaScript
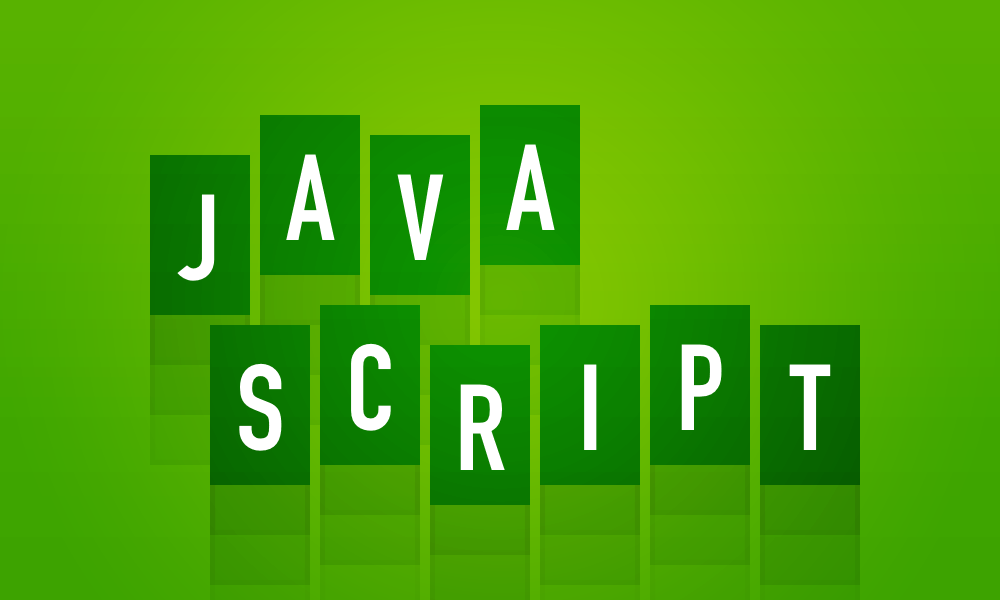
I came to Drupal from a JavaScript background and was happy to see a module system in place. Have you ever wished you could write your JavaScript modularly just like your PHP in order to take advantage of testing, better documentation and the Do One Thing Well philosophy? Well I have the solution for you: Browserify and NPM!
Getting StartedIn order to take advantage of JavaScript modularity you will need node and npm which can be installed together using one of these methods.
Creating a JavaScript ModuleJavaScript modules are similar to the PHP include system in that they allow the developer to separate their code across multiple files. The key difference is that JavaScript uses the syntax require to pull the exported part of a JavaScript file into the current file's variable of choice. Let's take a look.
Simply create a JavaScript file, do whatever you’re trying to do and assign the resulting value (usually a class or object) to module.exports.
// src/lib/MyClass.js module.exports = function MyClass(opts) {} MyClass.prototype.magic = function() { console.log(‘magic’); }You can now use MyClass.js from other JavaScript files like so:
// src/index.js var MyClass = require(‘./lib/MyClass’); var classy = new MyClass(); classy.magic(); // ‘magic’ NPMPHP developers may be familiar with Composer. NPM is the Composer of the JavaScript world. Both allow you to manage the dependencies of your project.
Before creating a custom module it's a great idea to see if it has already been written. npmjs.org is the place to look. When you find a module you’d like to include in your project, retrieve it and add it as a dependency with npm. For example, to install lodash:
npm install lodash --saveThe save flag adds this dependency to the package.json file. It might help to think of this as the JavaScript version of composer.json. All the dependencies for a project can be installed by running npm install within the directory containing package.json or its sub-directories.
Requiring modules from NPM is done just like local modules but you don’t have to provide the path, just use the module name. require will look in the node_modules folder automatically.
var _ = require(‘lodash’);Read more about NPM and package.json on the NPM website.
The Browserify commandBrowserify reads your JavaScript files and resolves their module.exports and require's.
Your main JavaScript file should be a compilation of its dependencies resulting in a useful piece of functionality for your website. To compile your multiple files into one that is browser ready we will use the browserify CLI.
First install it globally with npm. Globally installed modules can be accessed from anywhere on your machine.
npm install -g browserifyThen tell Browserify where your main JavaScript file is and redirect the output to where you want your compiled, browser-ready JS to be.
browserify src/index.js > build/myModule.jsbuild/myModule.js is now browser ready! Now you can add it to your website.
Come see me at BADCampThe above workflow is a great way to maintain custom Drupal modules with a lot of JavaScript. For more detailed information about using this workflow with Drupal — such as global dependencies, handling jQuery, task automation and testing — come check out my session at this year’s BADCamp!