Software
Blue Drop Shop: Update: Drupal Camp A/V Kit REBOOT!
In my initial test of a new session recording kit, some records were lost due to lack of audio. Also, the test setup used powered lav mics, which don't fly too well with multiple presenters.
As a follow up, I tested the Zoom H2N digital voice recorder because it just so happens to have a line out jack. So the question was whether that line out would be compatible with the HD PVR for audio. I'm happy to report that it is!
This is fantastic news for many reasons:
- Co-presenters or panels: Standing several feet away from the unit for the test resulted in great sound quality
- No microphone cords: Speakers are free to roam, if that is their style
- Redundancy: If the voice recorder is powered and hooked up correctly, the PVR will spit out a finished MP4, but should that audio fail for any reason, there will be a backup record on an SD card
At $160, the recorder definitely costs more than the original lav mic tested at DrupalCamp Fox Valley. With the suggested accessories (A/C power, tripod, wired remote, case, 32 MB SD Card, audio cable) the audio component comes up to about $225. This brings the total kit cost to just approximately $425 per room, which should accept a full day of recording and accommodate most laptops.
I'll be attending BADCamp and plan to bring the full kit with me, if anyone wants to check it out. Hell, if I get the chance, I will try to test it in the wild. Next steps are testing dongles for various portable devices, as well as contacting the Drupal Association to see what is needed to make these available for camps.
Huzzah!
Tags:Open Source Training: 7 Things to Know about the Drupal Security Issue
By now you've probably heard about the extremely serious Drupal security issue from mid-October.
The Drupal security team issued a new warning two weeks later that, if possible, escalated the severity of the issue.
Here's an overview of the issue and its impact.
Modules Unraveled: Why doesn't Drupal offer an auto update feature like Wordpress?
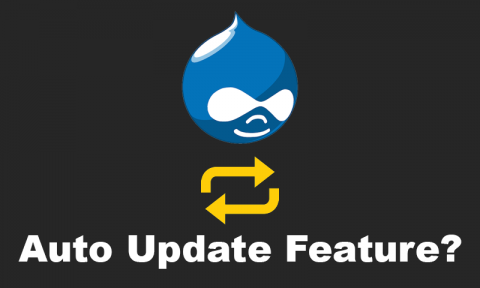
Let me start out by stating that I don't know the technical implications of an autocomplete feature. Okay? I don't have the answer. I'm just looking for information. Best case, I can help get something started that will benefit the entire Drupal community in the future.
With that out of the way, I firmly believe that anything is possible with Drupal. And with the "Drupageddon" of late, an auto update feature would be greatly appreciated by many, I'm sure. (I certainly would have benefited from one.)
I was recently discussing the security update with some friends, and one of them asked "Does Drupal have an auto update?"
And I was like "...no."
Immediately, I thought about all of the updates I don't immediately apply to contrib projects because they change a configuration option, or otherwise modify the way I've set up the site.
So I thought "I don't really want an auto update because it might break things.
That said, why can't Drupal automatically update security fixes - at least in core - automatically? If it did, Drupageddon could have never been a widespread issue.
It's easy to think, "Well, it's fixed now, so there's nothing to worry about."
But I think that's shortsighted.
The particular vulnerability that caused "Drupageddon" has been around since the inception of Drupal 7, which was officially released in 2011. So, for at least 3 years, every time we've fixed a security flaw, we've thought, "It's fixed now, so there's nothing to worry about." ... until the next issue was found, and this last one was a pretty gigantic one!
Wordpress introduced auto update in version 3.7 on October 24, 2013. They also included options in their configuration file that can be set to disable auto updates, as well as choose which types of updates should be performed automatically: none, major and minor or minor only.
You can read more about it on the Configuring Automatic Background Updates page:
http://codex.wordpress.org/Configuring_Automatic_Background_Updates
I'm just curious if this is something that can be added in a point release of D8 (like 8.5 or something).
Also, I've ready a few posts saying that auto updates would not fit their workflow. They use Drush, Git, etc. to manage their development workflow. And if that's you, I'd say that turning the auto update setting to off would mean that you can continue to work the way you currently do.
However, small business owners, churches, non-profits and the like that have volunteers (with little to no development background) managing their sites don't have the luxury of utilizing Git, Drush etc. In those scenarios, I think the case could be made that an autoupdate feature (as long as the updates are tested before release) could be a much more stable way of maintaining a site than having a volunteer FTP files to a server without really knowing what they are doing.
If you have thoughts, please add them below. I'd love to hear them!
Updates
- After doing some more research, I've found that some people tried to do this in D7, but postponed to D8. However, there hasn't been any movement since April 28, 2013. https://www.drupal.org/node/606592
- There's also a post explaining why auto updates would be a very bad idea from September 1, 2011. http://www.freelock.com/blog/john-locke/2011-09/why-auto-updates-are-very-bad-idea I'm not sure that I agree with everything he says though.
Bryan Ruby: Drupal Security: Not Shocking but Responsible
Over the years, I've made it an unwritten policy not to sensationalize bug fixes and security vulnerabilities in content management systems. While there may be great interest in such stories, I believe such stories have a tendency to cause more harm than good. When sensationalized, such articles tend to cause customers to address security concerns with emotion instead of logic which is never a good thing. So, when the security vulnerability known as "Drupageddon" broke and Drupal developer Bevan Rudge posted "Your Drupal website has a backdoor", I knew this story was going to eventually reach mainstream media. In the meantime, I've been struggling on how best to write this article and what story need to be told.
For those that don't know, Drupageddon is the highly critical SQL injection vulnerability in Drupal 7 core and was fully disclosed by the Drupal Security Team in SA-CORE-2014-005. Since the dawn of time when databases were introduced to websites, SQL injection vulnerabilities have been discovered and in the majority of cases when found are patched by their developers and system administrators. What makes Drupageddon particularly nasty is the vulnerability can be exploited by users not even logged into your site (in Drupal they're called anonymous users). Worse, if you didn't update your site quickly enough, your site may still be compromised even after applying the fix (in Drupal 7.32 or later versions).
It took two weeks, but the media have finally begun to use this Drupal event to sell their headlines. A recent BBC article claims that "up to 12 million websites may have been compromised by attackers who took advantage of a bug in the widely used Drupal software". While there is the potential for every single Drupal site on this earth to be compromised, I tend to believe Bevan Rudge's assessment that the real world numbers are more likely in the "hundreds of thousands". But the author of the article also found someone to state that this vulnerability and the need to audit your system for additional vulnerabilities is "shocking".
Having managed various software applications and websites for two decades, I find myself annoyed and angry that once again I'm patching and auditing my websites with extreme effort. We've all seen these type of security exploits in a wide range of software applications from a wide range of software developers. Ten years ago I discovered an ecommerce website that I managed hacked due to a SQL injection exploit. What upset me the most wasn't that the site was hacked but that the application's developers were aware of the problem for months but failed to publicly disclose the information to users. While the software industry has gotten better to disclose vulnerabilities and provide fixes for their software there is a lot of improvement than can still be made.
Perhaps what is shocking for those that don't know Drupal's open source community isn't the security exploit itself, but observing Drupal's willingness to fully disclose and take responsible steps to fix what is broken. It has been my experience that too many software vendors attempt to "soften the blow" in their disclosures to please the marketing arm of their company no matter how serious the exploit. Drupal on the other hand often takes the opposite approach. As a CMS critic I don't think I could write stronger words of warning in an article than what Drupal's community already does.
Drupal Security Team: A vulnerability in this API allows an attacker to send specially crafted requests resulting in arbitrary SQL execution. Depending on the content of the requests this can lead to privilege escalation, arbitrary PHP execution, or other attacks. This vulnerability can be exploited by anonymous users. [October 15, 2014]
Bevan Rudge, Drupal.Geek.NZ: I estimate hundreds of thousands of Drupal websites now have backdoors; between ten and ninety percent of all Drupal websites. Automated Drupageddon exploits were in the wild within hours of the announcement. Updating or patching Drupal does not fix backdoors that attackers installed before updating or patching Drupal. Backdoors give attackers admin access and allow arbitrary PHP execution.
If your Drupal 7 (and 8) website is not updated or patched it is most likely compromised. If your website was not updated within a day of the announcement, it is probably compromised. Even if your website was updated within a day, it may be compromised. [October 22, 2014]
Drupal Security Team: While recovery without restoring from backup may be possible, this is not advised because backdoors can be extremely difficult to find. The recommendation is to restore from backup or rebuild from scratch. [October 29, 1014]
I'm not a software developer, but I understand the news cycle for covering content management systems very well. Although this is a two week story for the Drupal community, we can expect to see more articles from authors and experts claiming their shock and dismay that such vulnerabilities in the Drupal software can exist. My spin is simply this, the media is only aware of this story because Drupal takes ownership and responsibility to disclose and address security issues in its own software. I personally find news of the vulnerability a non-story. The real story out there are the companies and software developers pointing fingers at Drupal and are not so forthcoming with their own security vulnerabilities. Those are the stories that need to be told.
This article was originally posted on CMS Report.
Tags:Károly Négyesi: MongoDB and Drupal 8: what and why?
Now that we have a fairly good idea how Drupal 8 and data looks let's discuss what can MongoDB provide and why would you want to run it. In Drupal 8, every kind of data can be stored independently. I fully expect that people will indeed mix storages. For example, D8 by default runs a config query on every page to find the blocks to be displayed for the current theme. Again, by default, config entities are stored as serialized PHP arrays so the only way a query like that can run is to load every single block entity from the database and iterate over them. This can get quite slow with hundreds of blocks -- don't forget that a block entity just stores the placement of a block and the same block can be placed several times. Also, after finding the blocks for the current theme, visibility rules (path, node types, roles) are applied again in PHP. If the configuration is stored in MongoDB then all this can become an indexed, practically instant query. Configuration storage, after all, is just storing and querying and retrieving arrays and MongoDB is really, really good at that. Because of this, I expect many sites to pick MongoDB for their configuration storage. I also expect that because of the simplicity of cross-entity type JOINs, many people will stick with SQL for their content storage. Although it must be noted that the choice can be made per entity type and as MongoDB stores complete entities it is able to index even those queries that the SQL storage can not.
There are some simpler storages which are also a good fit for MongoDB: sessions because of the write performance and logging because MongoDB has a capped collection (a circular buffer) feature so you will always have the latest N messages and never too much.
Paul Booker: 5 commands that help with drupalgeddon
Showing files that have changed on the live server:
git statusLooking for code execution attempts via menu_router:
select * from menu_router where access_callback = 'file_put_contents'Showing which files are on the live server and not in version control:
diff -r docroot repo | grep docroot | grep 'Only in docroot'Finding PHP files in the files directory:
find . -path "*php"Checking the amount of time between when a user logged into your site and their most recent page visit:
select (s.timestamp - u.login) / 60 / 60 / 24 AS days_since_login, u.uid from sessions s inner join users u on s.uid = u.uid;Hotfix: (SA-CORE-2014-005)
curl https://www.drupal.org/files/issues/SA-CORE-2014-005-D7.patch | patch -p1Sorry , that was 6. Please add others in the comments.
If you need help regarding the recent drupal vulnerability feel free to contact me.
Tags: TweetStauffer: Drupal Internationalization
Drupal core announcements: What changes are allowed during the Drupal 8 beta phase?
Now that Drupal 8 is in beta, we are narrowing the changes we allow to Drupal 8 core to accelerate our progress toward Drupal 8's release. The Drupal 8 branch maintainers have established a policy on the allowed changes during the Drupal 8 beta to help contributors understand what changes are no longer allowed. All core contributors should review this policy and try to apply it in each issue.
Key updates for core contributors- To take full advantage of the sprints at DrupalCon Amsterdam, we allowed one month after the initial beta release for many changes to go in. The deadline for those issues was October 27, so now all issues are subject to the beta policy.
- Many changes will now be postponed to a later release, especially many types of normal tasks that do not directly help make Drupal 8 releasable. See the policy issue for specifics.
- We will also be more rigorous about issue priority settings. For example, many issues that are currently major tasks will be reassessed and possibly downgraded to normal (and subsequently may be postponed).
See the beta changes policy for details.
Read over the new policy, and take special note of how to evaluate issues. Help by posting on your issues with where they fall under the policy.
Acquia: Acquia’s Response to the October 15 Drupal Security Alert
Acquia is committed to ensuring the security and performance of our customers’ sites.
Appnovation Technologies: AJAXify Your Links
You can apply AJAX to any elements on the page by adding the use-ajax class to that element. Typically we apply this to link elements.

Chris Hall on Drupal 8: Drupageddon a chance to prove the calibre of Drupal community
I doubt that many people who read this will be unaware of the extremely severe security vulnerability that was discovered reported and patched a couple of weeks ago or the later realease and many related blog posts pointing out exactly how critical early updates and patching are.
If this has ruined a little of your free time recently and/or entailed your agency really earning the costs of any maintenance contracts you offer consider how terrifying some of the press and implications are for owners of unsupported Drupal sites many of whom will be small charities and local organisations.
I would like to think that local Drupal groups etc. and the 'Drupal community' in general would step up and help out, if enough of us do that then we could generate some positive press. Yes we have a security team etc that is good, but how are we going to help out?
My local Drupal group will attempt to answer questions and find people to provide a little support (anybody else??),
Appreciate that many people are not in the position to provide a lot of effort for free, even a small amount of advice could get people on the right track and Drupal groups are likely to know good freelancers that can afford to help a small company for considerably less than typical agency fees.
All those hackthons, sprints, efforts to drive D8 forward, anybody brave enough to divert some of that effort towards auditing/fixing local sites?? I hope so.
BTW I have slight doubts about this site although I did fix by hand based on this commit (this is an old alpha). I will be trashing this server shortly and migrating to a new Beta 2 site and fresh server.
Tags:
- planet
Acquia: Acquia, Investors and Teaming Up to Build Greatness
In the summer of 2007, I received an email from Michael Skok of Northbridge Venture Partners, who I had known for 12 years at that time. I had recently exited Mercury Interactive after its acquisition by HP. He suggested that I meet with a entrepreneur around a project he was looking at. When I met with Jay Batson, and short thereafter with Dries Buytaert, I was intrigued. It was Michael's vision about the possibilities however that really grabbed my attention. The three asked me later that year to join the yet unnamed company as its founding CEO.
Lullabot: The Front-end Rapport
In this episode Kyle is joined by a few members of Lullabot's front-end army.
Drupal Bits at Web-Dev: Intro to Codit Crons - Cron Jobs made easy.
This is a video introduction to the Drupal module Codit: Crons.
Codit: Crons uses the Codit framework to make the development of multiple cron jobs easy. In most cases it ends up being both more flexible, and safer than custom coding hook_cron() in a custom module or Feature for a site.
Why should I consider using Codit: Crons? Continue reading Codit: Crons IntroductionThinkShout: It's a Good Time to Go to BADCamp
It’s that time of year again! And boy, are we excited... BADCamp is around the corner and we’ve already got our bags packed for San Francisco. BADCamp is one of our favorite Drupal Camps out there because it’s close to home, attendance is free, and it offers a handful of great Drupal trainings for all skill levels. And, of course, there’s the Nonprofit Summit that takes place on Thursday, November 6.
We’ve been hard at work helping coordinate this summit and we’re thrilled with the day that’s come together.
We’ve lined up speakers from the Sierra Club, Kiva, and the Electronic Frontier Foundation, who will share their experience and insights into leveraging Drupal to further their mission. You’ll also be able to join a variety of discussion-style breakout sessions, led by Drupal experts and nonprofit tech leaders. Looking for a topic that’s not on the schedule? Lead a discussion of your own during the open breakout sessions!
We’ve got a jampacked schedule in addition to the Nonprofit Summit day. On Saturday and Sunday, you can find ThinkShout at booth #4 - stop by and say hi - we’d love to chat with our fellow BADCampers. Several members of our team will be speaking - check out the schedule below for a full breakdown of our presentations.
Saturday, Nov. 8
"Maps Made Easy" - Gabe Carleton-Barnes (@uncle_gcb)
Room: Rainbow Road
Time: 10:00am-11:00am
Maps are all over the web these days, and they can be extremely effective tools for finding and sharing information. Embedding a simple Google Map is easy, but what about building something that is more integrated with your content? It turns out you can build awesome integrated maps in Drupal almost entirely with point-and-click tools: all you need is the Open Source Leaflet library, Views, and a few other Contrib Modules. In this session we'll show you how by interactively building a site with a map from a bare Drupal 7 install.
Participants will choose what type of mappable content to create, and will be asked to add content using their own devices to build a rich demonstration of the map's capabilities.
As we assemble the required modules and configure our site, we will discuss the roles played by each module without a bunch of geospatial techno-babble. By the end of the session, we will have an interactive map displaying content that is easy for any site user to input using address data. If there is sufficient time, we will discuss how to customize the map's "tiles", add plugins, use proximity filtration, and other potential features for your map!
"Responding to Responsive - A Designer’s Guide to Adapting" - Josh Riggs (@joshriggs)
Room: Warp Zone
Time: 10:00am-10:30am
There’s no denying that a designer’s role is changing. Responsive Design has made the whole process much more complex. Designers are now expected to be equal parts artist and coder, and to use HTML, CSS & Javascript as their palette. I’ve met that challenge, and I‘ve spent the last few years working on several large, responsive Drupal sites. This talk will include a candid, real-world look at my personal evolving design process, as well as lessons from my own personal journey as a designer.
Topics include:
A thorough walkthrough of responsive design deliverables: Content Strategy, HTMLWireframes, Style Tiles, & Style Mocks. Examples will be shown.
Managing the expectations of Clients, Users and Developers
Keeping the focus on User Experience
Real world successes and failures
A fight to the death between Photoshop & In-Browser design
Creating a better iterative process
Bridging the gap between design and front-end development
Embracing the concept of Kaizen (continuous improvement) as a designer
"Responsive Image Loading with the Picture Module"- Cooper Stimson (@cooperstimson)
Room: Warp Zone
Time: 5:30pm-6:00pm
Responsive Web Design (RWD) is increasingly vital in the contemporary web landscape, where your content can be displayed on a phone, a laptop, an 84-inch 4k monitor, a refrigerator, or even a watch. In this session you will learn how to leverage the Picture module (and its dependency, the Breakpoints module) to achieve responsive image loading in Drupal 7.
The Picture Module
There is no RWD solution for images in Drupal 7 core. Luckily, a responsive image handling module called Picture will be included in Drupal 8 core, and has already been backported to Drupal 7. Picture uses the new HTML5 picture element.
This session will cover:
Installation and configuration of the Picture, Media, Chaos Tools Suite, and Breakpoint modules
Creating breakpoints and breakpoint groups
Configuring picture mappings
Setting up file type display settings
Applying these options to an example content type
Basic introduction to the picture element and media queries
Image loading is particularly important for RWD; loading an image size inappropriate to screen resolution is problematic whether you're stretching a 100x100 thumbnail over a massive screen, or sending a ten megapixel background to a QVGA phone. In the former case you're making a pixelated mess, and in the latter case you're eating up both your own bandwidth and your user's data plan on a resolution they can't use - nobody wins.
Benefits for Your Site
There are many reasons to responsively load images on your site. A few highlights are:
Consistent user experience across platforms
Single URL per page
- No need to code up a separate mobile version
- SEO optimization -- Don't split your pageview count between multiple URLs
- Improved shareability
Massive pageload benefits on mobile
Target Audience
This session is aimed at beginning level Drupalers who haven't used the Picture module before.
It’s completely free to register, just sign up on the BADCamp website.
See you in San Francisco!
Code Karate: Drupal 7 Publication Date Module

In this video we look at the Publication Date module. This module allows content creators to use the date in which the content on their website is published and NOT just when it is created. In other words, if you create a post a week prior to publishing it, this module will use the date in which the post goes to published. Again, this is a simple module but can be extremely helpful if you post a lot of content or have a habit of writing content days or weeks prior to publishing.
Tags: DrupalFieldsViewsDrupal 7Drupal PlanetMediacurrent: Why You Should Speak at Tech Conferences

The first time I spoke at a tech conference was about five years ago at the University of Southern California (USC), in Los Angeles. It was at an annual conference called Code Camp whose audience is mostly Microsoft developers. I didn’t know what to expect in that kind of setting. I selected a topic I was fairly comfortable with, Designing with CSS3. Not only was the topic well received but it quickly became the most popular session in the conference with over 140 attendees interested in it. Now I was really freaking out.
Chromatic: Easily Upgrade Your Image Fields for Retina!
Drupal makes it so easy to add image fields to your content types. Fields in core for the win! With image styles in core, its as easy as ever to create multiple image sizes for display in various contexts (thumbnails, full, etc.). But what about providing hi-resolution versions of your rasterized images for retina displays? Out of the box, you don’t really have a lot of good options. You could simply upload high resolution versions and force your users, regardless of display type to download massive file versions, but that’s not exactly the best for performance. You could use some custom field theming and roll your own implementation of the <picture> element, but browser support is basically null at this point. That won’t do. You could do what Apple does and force the browser to download 1x versions of your images then use javascript to detect high resolution displays and then force the browser to download all of the high resolution versions…I think you see my point.
What if you could create hi-resolution versions of these images without a ton of added filesize overhead? What if you could do this all within Drupal? No special coding, no uploading of multiple versions, no special field templates or unnecessary javascript. Just a basic Drupal image field with a special image style defined.
Here’s how you do it:- Create your image field. (In most cases, you’ve probably already got this.)
- Download and install the HiRes Images module This module allows you to create an image style at 2x the desired pixel dimensions. If your desired maximum image width is 720 css pixels, your output image would be saved at 1440px.
- Download and install the Image Style Quality module This nifty module allows you to define specific image qualities on a per image style basis instead of using Drupal’s global image quality setting.
- Add a new image style (or alter an existing)
- Add your normal image style presets, like scale, crop etc. If you’re scaling, set your scale to be 2x your desired maximum output in pixels. So if you want an output of 720, set your scale to 1440px.
- Add the “Hi-Res (x2)”" effect. This will output you’re image element at half the scale amount above. So we get a max of 720px.
- Add the “Quality” effect and set it to something like 60%. This may take some experimenting to find a balance between image quality and file size. In my example, I went with 60% compression. This yielded an image that was still really sharp and a reasonable file size.
- Set your display mode to use this new (or altered) image style.
- Enjoy your beautiful, high resolution, performant image fields!
Hard to believe this works right? You’d think your retina version would look really crappy with that much compression, but it doesn’t. In fact, in some cases it will look just as sharp and be smaller than a 1x counterpart. See my screenshots below for proof:
Side-by-side comparison: Network panel output:So we end up with a high resolution version of our uploaded image that is actually smaller than the original version at 720px! Looks great on retina devices and doesn’t badly penalize users of standard definition displays. WIN!
For a detailed explanation of this technique in broader terms, see Retina Revolution by Daan Jobsis
Midwestern Mac, LLC: How to set complex string variables with Drush vset
I recently ran into an issue where drush vset was not setting a string variable (in this case, a time period that would be used in strtotime()) correctly:
# Didn't work:$ drush vset custom_past_time '-1 day'
Unknown options: --0, --w, --e, --k. See `drush help variable-set` [error]
for available options. To suppress this error, add the option
--strict=0.
Using the --strict=0 option resulted in the variable being set to a value of "1".
After scratching my head a bit, trying different ways of escaping the string value, using single and double quotes, etc., I finally realized I could just use variable_set() with drush's php-eval command (shortcut ev):
Jonathan Brown: Update on Drupal / Bitcoin Payment Protocol (BIP 70) integration
BIP 70 describes a high-level payment system for Bitcoin. It uses Protocol Buffers and X.509 certificates for the following major improvements:
- Human-readable payment destinations instead of Bitcoin addresses
- Resistance from man-in-the-middle attacks
- Payment received messages sent back to the wallet
- Refund addresses
I compiled the BIP 70 Protocol Buffers definition file into PHP using ProtobufPHP.
I have implemented most of BIP 70 in the Coin Tools Drupal project. It contains a new Bitcoin payment entity class that contains all the specified fields in its base table. Bundles can be created to add additional fields to payments.
Payments can currently be created through an admin interface, although this would typically happen in an automated process on a real website.
When viewing an unfulfilled payment in the admin interface the QR code for the payment will be present. It decodes to a backward-compatible payment protocol URI.
Currently the module is unable to detect Bitcoin payments not sent using the payment protocol, i.e. the payment is sent to the address but the website is not notified. This will be quite easy to implement though.
For payments made using the new protocol, Coin Tools is able to complete the transaction and has been tested with both the original QT client and Andreas Schildbach's Android Wallet. Interestingly Andreas's wallet does not display the status message returned by the merchant.
The specification does not seem to have any method for the merchant to inform the app that the payment was not satisfactory, other than setting the human readable status message (the wallet would not know there was a problem), or returning an HTTP error code (resulting in unpleasant error message for customer).
Coin Tools will check the transactions provided by the wallet are sending enough bitcoins to the payment address. It then broadcasts the transactions via bitcoind. Currently Coin Tools is relying on bitcoind rejecting transactions that have not been signed correctly. This assumption needs to be verified.
When the payment protocol QR code is displayed, Coin Tools enables a small Javascript program to poll the website to determine if the payment has been made, reloading the page once this has happened. Ideally this would be implemented as a long-running AJAX request.
The X.509 certificate part of the payment protocol specification has not yet been implemented in Coin Tools. This is a critical component.
The implementation of the payment protocol in Coin Tools only permits a single Bitcoin address per payment. The specification does support having more than one and in theory this could be used to increase payment anonymity by each address only being spent into by a single output in a single transaction. In practise this is not so effective as all the transactions would be broadcast simultaneously.
Coin Tools will also store a single refund address provided by the wallet making the payment. The wallet actually provides payment scripts, but Coin Tools will determine if the script is a standard payment and extract the address. Multiple refund addresses are also supported by the standard, but Coin Tools will only store one.
According to the specification the wallet can allow the customer to provide a note to the vendor. Coin Tools will store this note, however I do not know of any wallets that support this feature.
The HTTP responses for PaymentRequest and Payment need to be implemented as Symfony response handlers. Currently they are implemented in a simplistic manner setting their own HTTP headers and and calling exit().
It is currently only possible to make payments from the admin interface. A template needs to be provided so the payments can be made from elsewhere on a website, e.g. integration with Drupal Commerce.
For a standard ecommerce website that wants to accept bitcoins it may make more sense to use a provider such as BitPay or Coinbase. Accepting payments natively on a website means that a hacker could steal funds. One solution to this problem would be to use Hierarchical Deterministic Wallets so that private keys are only stored on backend systems.
For a project that is doing something more interesting than just accepting Bitcoin as a payment method and is already running bitcoind, it may be advantageous to have a native implementation on BIP 70 on the website rather than relying on a third-party provider.
No tests have yet been written for Coin Tools. It is essential that Payment and PaymentRequest routes are fully tested including the edge cases defined in the specification.
A few limitations of Drupal 8 have been encountered during the creation of this functionality. In Drupal 8 it is now possible to have fields in entity base tables. This is really great, but unfortunately when these fields are present in a view it is not possible to use their formatters. I discussed this with Daniel Wehner at Amsterdam and he didn't seem very optimistic about this being able to be fixed so some sort of workaround will need to be found as this functionality is critical to the module.
Date field is now in D8 core, but unfortunately it stores the date as a varchar in the database. This means that it is not possible to sort or filter on date - a major limitation. If core is not changed to use database-native date storage Coin Tools will have to use another date field.
The Payment Protocol functionality needs to be backported to Drupal 7 Coin Tools and integrated with Payment / Commerce.