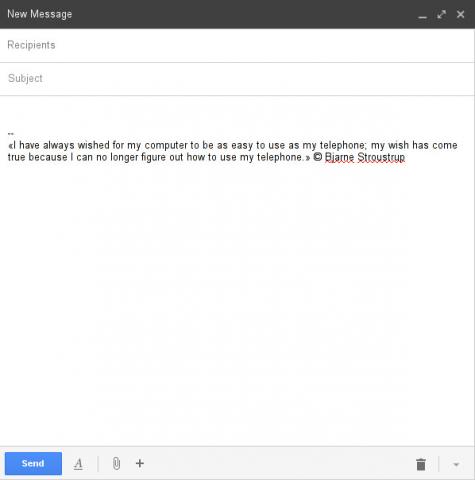
It almost works. It will update signatures that you store in a Google Sheet at regular intervals. The only problem is… You have to be a Google Apps for Business administrator to run this thing, because the only way to update a Gmail signature programmatically is via the Google Apps for Business Admin SDK. Ha-ha. Another problem is that the signature only gets updated when you refresh the page. But that's a minor thing ;-)
Check it out and make a copy to have access to the script.. And here's the code:
function onOpen() { var ss = SpreadsheetApp.getActive(); ss.addMenu("FortunKa", [{name: "Configure", functionName: "doGet"}]); } function doGet() { var app = UiApp.createApplication(); var handler = app.createServerHandler("onSave"); var panel = app.createVerticalPanel(); var email = app.createTextBox().setName("email").setValue(UserProperties.getProperty("email")); var passwd = app.createPasswordTextBox().setName("passwd").setValue(UserProperties.getProperty("passwd")); var domain = app.createTextBox().setName("domain").setValue(UserProperties.getProperty("domain")); var login = app.createTextBox().setName("login").setValue(UserProperties.getProperty("login")); var button = app.createButton("Save").addClickHandler(handler); var explanation = app.createLabel("The fortune generator works only for Google Apps for Business administrators." + "The administrator email and password are needed to create an authentication " + "token for Google Apps for Business Email Setting API. The domain is the Google " + "Apps for Business domain. The login is the login of the user whose signature will " + "be changed. " + "To set up regular signature updates, go to Resources / Current" + "project triggers and setup a time-driven task using the run function."); var grid = app.createGrid(4, 2); grid.setWidget(0, 0, app.createLabel("Administrator email:")); grid.setWidget(0, 1, email); grid.setWidget(1, 0, app.createLabel("Administrator Password:")); grid.setWidget(1, 1, passwd); grid.setWidget(2, 0, app.createLabel("Domain name:")); grid.setWidget(2, 1, domain); grid.setWidget(3, 0, app.createLabel("User login:")); grid.setWidget(3, 1, login); var panel = app.createVerticalPanel().add(grid).add(button).add(explanation); handler.addCallbackElement(panel); app.add(panel); var spreadsheet = SpreadsheetApp.getActiveSpreadsheet(); spreadsheet.show(app); } function onSave(e) { UserProperties.setProperty("email", e.parameter.email); UserProperties.setProperty("passwd", e.parameter.passwd); UserProperties.setProperty("domain", e.parameter.domain); UserProperties.setProperty("login", e.parameter.login); var app = UiApp.getActiveApplication(); app.close(); return app; } function getToken(email, passwd) { var payload = { "Email" : email, "Passwd" : passwd, "accountType": "HOSTED", "service": "apps" }; var params = { "method" : "post", "payload" : payload }; var response = UrlFetchApp.fetch('https://www.google.com/accounts/ClientLogin', params); if (response.getResponseCode() == 200) { //Logger.log(response.getContentText().match(/Auth=(.*)/)[1]); return response.getContentText().match(/Auth=(.*)/)[1]; } } /* * PUT https://apps-apis.google.com/a/feeds/emailsettings/2.0/example.com/liz/signature <?xml version="1.0" encoding="utf-8"?> <atom:entry xmlns:atom="http://www.w3.org/2005/Atom" xmlns:apps="http://schemas.google.com/apps/2006"> <apps:property name="signature" value="Liz Jones - (+1) 619-555-5555 Accounts Management, A&Z LTD." /> </atom:entry> */ function updateSignature(token, domain, login, fortune){ var atom = XmlService.getNamespace("atom", "http://www.w3.org/2005/Atom"); var apps = XmlService.getNamespace("apps","http://schemas.google.com/apps/2006"); var entry = XmlService.createElement("entry", atom); var property = XmlService.createElement("property", apps) .setAttribute("name", "signature") .setAttribute("value", fortune); entry.addContent(property); var document = XmlService.createDocument(entry); var xml = XmlService.getPrettyFormat().format(document); //Logger.log(xml); var headers = { "Content-type": "application/atom+xml", "Authorization": "GoogleLogin auth=" + token }; var params = { "method" : "put", "headers" : headers, "payload": xml }; var response = UrlFetchApp.fetch("https://apps-apis.google.com/a/feeds/emailsettings/2.0/" + domain + "/" + login + "/signature", params); Logger.log(response.getResponseCode()); Logger.log(response.getContentText()); } function getFortune() { var sheet = SpreadsheetApp.getActiveSheet(); var data = sheet.getDataRange().getValues(); var random = getRandom(0,data.length); data[random][0] return data[random][0]; } function getRandom(min, max) { return Math.floor(Math.random() * (max - min + 1) + min); } function run(){ var email = UserProperties.getProperty("email"); var passwd = UserProperties.getProperty("passwd"); var domain = UserProperties.getProperty("domain"); var login = UserProperties.getProperty("login"); if (email && passwd && domain && login) { var fortune = getFortune(); updateSignature(getToken(email, passwd), domain, login, fortune); } }
Tags: